svgui
1.9
|
SpectrumLayer.h
Go to the documentation of this file.
virtual void setProperty(const PropertyName &, int value) override
Definition: SpectrumLayer.cpp:267
virtual ModelId getModel() const override
Return the ID of the model represented in this layer.
Definition: SpectrumLayer.h:41
virtual void getBiasCurve(BiasCurve &) const override
Definition: SpectrumLayer.cpp:938
bool needsTextLabelHeight() const override
True if this layer will need to place text labels when it is painted.
Definition: SpectrumLayer.h:104
Definition: Layer.h:141
virtual RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: SpectrumLayer.cpp:261
virtual QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: SpectrumLayer.cpp:638
Definition: SliceLayer.h:27
virtual double getXForBin(const LayerGeometryProvider *, double bin) const override
Convert a (possibly non-integral) bin into x-coord. May be overridden.
Definition: SpectrumLayer.cpp:432
virtual double getXForFrequency(const LayerGeometryProvider *, double freq) const override
Definition: SpectrumLayer.cpp:442
virtual void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: SpectrumLayer.cpp:732
Definition: SpectrumLayer.h:31
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
virtual int getHorizontalScaleHeight(LayerGeometryProvider *, QPainter &) const override
Definition: SpectrumLayer.cpp:877
void preferenceChanged(PropertyContainer::PropertyName name)
Definition: SpectrumLayer.cpp:366
virtual QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SpectrumLayer.cpp:231
virtual double getFrequencyForX(const LayerGeometryProvider *, double x) const override
Definition: SpectrumLayer.cpp:408
virtual bool getYScaleValue(const LayerGeometryProvider *, int y, double &value, QString &unit) const override
Return the value and unit at the given y coordinate in the given view.
Definition: SpectrumLayer.cpp:465
virtual int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SpectrumLayer.cpp:180
virtual VerticalPosition getPreferredFrameCountPosition() const override
Definition: SpectrumLayer.h:54
virtual bool getYScaleDifference(const LayerGeometryProvider *, int y0, int y1, double &diff, QString &unit) const override
Return the difference between the values at the given y coordinates in the given view, and the unit of the difference.
Definition: SpectrumLayer.cpp:491
virtual PropertyType getPropertyType(const PropertyName &) const override
Definition: SpectrumLayer.cpp:160
virtual double getFrequencyForBin(double bin) const
Definition: SpectrumLayer.cpp:422
virtual double getBinForX(const LayerGeometryProvider *, double x) const override
Convert an x-coord into (possibly non-integral) bin. May be overridden.
Definition: SpectrumLayer.cpp:398
virtual void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SpectrumLayer.cpp:944
virtual bool setDisplayExtents(double min, double max) override
Set the displayed minimum and maximum values for the y axis to the given range, if supported...
Definition: SpectrumLayer.cpp:378
virtual void paintCrosshairs(LayerGeometryProvider *, QPainter &, QPoint) const override
Definition: SpectrumLayer.cpp:548
virtual void paintHorizontalScale(LayerGeometryProvider *, QPainter &, int xorigin) const
Definition: SpectrumLayer.cpp:889
virtual PropertyList getProperties() const override
Definition: SpectrumLayer.cpp:132
void setOversampling(int oversampling)
Definition: SpectrumLayer.cpp:326
virtual bool getXScaleValue(const LayerGeometryProvider *v, int x, double &value, QString &unit) const override
Return the value and unit at the given x coordinate in the given view.
Definition: SpectrumLayer.cpp:456
virtual double getBinForFrequency(double freq) const
Definition: SpectrumLayer.cpp:388
virtual void setProperties(const QXmlAttributes &) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SpectrumLayer.cpp:960
virtual QString getPropertyGroupName(const PropertyName &) const override
Definition: SpectrumLayer.cpp:170
virtual QString getPropertyLabel(const PropertyName &) const override
Definition: SpectrumLayer.cpp:143
virtual bool getCrosshairExtents(LayerGeometryProvider *, QPainter &, QPoint cursorPos, std::vector< QRect > &extents) const override
Definition: SpectrumLayer.cpp:501
virtual bool isLayerScrollable(const LayerGeometryProvider *) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: SpectrumLayer.h:82
virtual QString getPropertyIconName(const PropertyName &) const override
Definition: SpectrumLayer.cpp:153
Interface to be implemented by objects, such as layers or objects they delegate to, in which the X axis corresponds to frequency.
Definition: HorizontalScaleProvider.h:26
Generated by
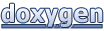