svgui
1.9
|
ColourScale.cpp
Go to the documentation of this file.
Definition: ColourScale.h:37
double threshold
Threshold below which every value is mapped to background pixel 0.
Definition: ColourScale.h:59
QColor getColourForPixel(int pixel, int rotation) const
Return the colour for the given pixel number (which must be in the range 0-255).
Definition: ColourScale.cpp:157
ColourScale(Parameters parameters)
Create a ColourScale with the given parameters.
Definition: ColourScale.cpp:28
bool hasLightBackground() const
Return true if the colour map is intended to be placed over a light background, false otherwise...
Definition: ColourMapper.cpp:488
ColourScaleType getScale() const
Return the general type of scale this is.
Definition: ColourScale.cpp:96
int getPixel(double value) const
Return a pixel number (in the range 0-255 inclusive) corresponding to the given value.
Definition: ColourScale.cpp:102
Generated by
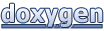