svgui
1.9
|
Colour3DPlotLayer.h
Go to the documentation of this file.
QString getPropertyValueIconName(const PropertyName &, int value) const override
Definition: Colour3DPlotLayer.cpp:480
bool getDisplayExtents(double &min, double &max) const override
Return the minimum and maximum values within the visible area for the y axis of this layer...
Definition: Colour3DPlotLayer.cpp:726
int getCurrentVerticalZoomStep() const override
Get the current vertical zoom step.
Definition: Colour3DPlotLayer.cpp:776
static std::pair< ColumnNormalization, bool > convertToColumnNorm(int value)
Definition: Colour3DPlotLayer.cpp:120
void invalidateRenderers()
Definition: Colour3DPlotLayer.cpp:201
static int convertFromColourScale(ColourScaleType)
Definition: Colour3DPlotLayer.cpp:105
ModelId getModel() const override
Return the ID of the model represented in this layer.
Definition: Colour3DPlotLayer.h:49
ModelId getExportModel(LayerGeometryProvider *) const override
Return the ID of a model representing the contents of this layer in a form suitable for export to a t...
Definition: Colour3DPlotLayer.cpp:220
PropertyType getPropertyType(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:329
QString getPropertyLabel(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:306
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: Colour3DPlotLayer.cpp:1218
std::map< int, Colour3DPlotRenderer * > ViewRendererMap
Definition: Colour3DPlotLayer.h:204
ModelId getSliceableModel() const override
Definition: Colour3DPlotLayer.h:155
void setVerticalZoomStep(int) override
Set the vertical zoom step.
Definition: Colour3DPlotLayer.cpp:787
void setProperty(const PropertyName &, int value) override
Definition: Colour3DPlotLayer.cpp:505
int getCompletion(LayerGeometryProvider *) const override
Return the proportion of background work complete in drawing this view, as a percentage – in most ca...
Definition: Colour3DPlotLayer.cpp:702
Definition: Layer.h:353
This is a view that displays dense 3-D data (time, some sort of binned y-axis range, value) as a colour plot with value mapped to colour range.
Definition: Colour3DPlotLayer.h:41
int getVerticalScaleWidth(LayerGeometryProvider *v, bool, QPainter &) const override
Definition: Colour3DPlotLayer.cpp:938
bool isLayerScrollable(const LayerGeometryProvider *v) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: Colour3DPlotLayer.cpp:693
double getYForBin(const LayerGeometryProvider *, double bin) const override
Return the y coordinate at which the given bin "starts" (i.e.
Definition: Colour3DPlotLayer.cpp:823
void handleModelChanged(ModelId)
Definition: Colour3DPlotLayer.cpp:256
void setInvertVertical(bool i)
Definition: Colour3DPlotLayer.cpp:617
bool getInvertVertical() const
Definition: Colour3DPlotLayer.cpp:644
std::map< int, MagnitudeRange > ViewMagMap
Definition: Colour3DPlotLayer.h:199
bool snapToFeatureFrame(LayerGeometryProvider *v, sv_frame_t &frame, int &resolution, SnapType snap, int ycoord) const override
Adjust the given frame to snap to the nearest feature, if possible.
Definition: Colour3DPlotLayer.cpp:1252
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
Definition: Colour3DPlotRenderer.h:49
void setGain(float gain)
Set the gain multiplier for sample values in this view.
Definition: Colour3DPlotLayer.cpp:552
Interface for layers in which the Y axis corresponds to bin number rather than scale value...
Definition: VerticalBinLayer.h:28
ColourSignificance getLayerColourSignificance() const override
This should return the degree of meaning associated with colour in this layer.
Definition: Colour3DPlotLayer.h:76
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: Colour3DPlotLayer.cpp:1280
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: Colour3DPlotLayer.cpp:360
ViewMagMap m_lastRenderedMags
Definition: Colour3DPlotLayer.h:201
static ColourScaleType convertToColourScale(int value)
Definition: Colour3DPlotLayer.cpp:93
void invalidateMagnitudes()
Definition: Colour3DPlotLayer.cpp:211
bool hasLightBackground() const override
Definition: Colour3DPlotLayer.cpp:662
void setSynchronousPainting(bool synchronous) override
Enable or disable synchronous painting.
Definition: Colour3DPlotLayer.cpp:147
int getVerticalZoomSteps(int &defaultStep) const override
Get the number of vertical zoom steps available for this layer.
Definition: Colour3DPlotLayer.cpp:765
QString getPropertyIconName(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:320
void setColourScale(ColourScaleType)
Definition: Colour3DPlotLayer.cpp:533
int getColourScaleWidth(QPainter &) const
Definition: Colour3DPlotLayer.cpp:930
ColourScaleType getColourScale() const
Definition: Colour3DPlotLayer.h:100
bool getNormalizeVisibleArea() const
Definition: Colour3DPlotLayer.cpp:611
PropertyList getProperties() const override
Definition: Colour3DPlotLayer.cpp:291
QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: Colour3DPlotLayer.cpp:865
void paintWithRenderer(LayerGeometryProvider *v, QPainter &paint, QRect rect) const
Definition: Colour3DPlotLayer.cpp:1163
const ZoomConstraint * getZoomConstraint() const override
Return a zoom constraint object defining the supported zoom levels for this layer.
Definition: Colour3DPlotLayer.cpp:85
void setNormalizeVisibleArea(bool)
Specify whether to normalize the visible area.
Definition: Colour3DPlotLayer.cpp:599
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
int getColourMap() const
void setProperties(const QXmlAttributes &) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: Colour3DPlotLayer.cpp:1332
void invalidatePeakCache()
Definition: Colour3DPlotLayer.cpp:188
bool m_normalizeVisibleArea
Definition: Colour3DPlotLayer.h:174
const int m_peakCacheDivisor
Definition: Colour3DPlotLayer.h:193
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: Colour3DPlotLayer.cpp:444
static int convertFromColumnNorm(ColumnNormalization norm, bool visible)
Definition: Colour3DPlotLayer.cpp:132
bool setDisplayExtents(double min, double max) override
Set the displayed minimum and maximum values for the y axis to the given range, if supported...
Definition: Colour3DPlotLayer.cpp:746
void paintVerticalScale(LayerGeometryProvider *v, bool, QPainter &paint, QRect rect) const override
Definition: Colour3DPlotLayer.cpp:966
ColumnNormalization getNormalization() const
Definition: Colour3DPlotLayer.cpp:593
RangeMapper * getNewVerticalZoomRangeMapper() const override
Create and return a range mapper for vertical zoom step values.
Definition: Colour3DPlotLayer.cpp:810
Colour3DPlotRenderer * getRenderer(const LayerGeometryProvider *) const
Definition: Colour3DPlotLayer.cpp:1090
void setLayerDormant(const LayerGeometryProvider *v, bool dormant) override
Indicate that a layer is not currently visible in the given view and is not expected to become visibl...
Definition: Colour3DPlotLayer.cpp:669
bool getYScaleValue(const LayerGeometryProvider *, int, double &, QString &) const override
Return the value and unit at the given y coordinate in the given view.
Definition: Colour3DPlotLayer.cpp:758
void handleModelChangedWithin(ModelId, sv_frame_t, sv_frame_t)
Definition: Colour3DPlotLayer.cpp:273
void setNormalization(ColumnNormalization)
Specify the normalization mode for individual columns.
Definition: Colour3DPlotLayer.cpp:582
bool getValueExtents(double &min, double &max, bool &logarithmic, QString &unit) const override
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: Colour3DPlotLayer.cpp:710
double getBinForY(const LayerGeometryProvider *, double y) const override
Return the bin number, possibly fractional, at the given y coordinate.
Definition: Colour3DPlotLayer.cpp:842
RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:496
ColumnNormalization m_normalization
Definition: Colour3DPlotLayer.h:173
QString getPropertyGroupName(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:340
Generated by
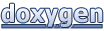