svgui
1.9
|
Colour3DPlotExporter.h
Go to the documentation of this file.
QString getTypeName() const override
Definition: Colour3DPlotExporter.h:114
ColumnNormalization normalization
Type of column normalization.
Definition: Colour3DPlotExporter.h:68
sv_samplerate_t getSampleRate() const override
Definition: Colour3DPlotExporter.h:107
const LayerGeometryProvider * provider
Definition: Colour3DPlotExporter.h:31
Definition: Colour3DPlotExporter.h:20
BinDisplay binDisplay
Selection of bins to include in the export.
Definition: Colour3DPlotExporter.h:46
double gain
Gain that is applied before thresholding, in the display, matching the ColourScale object parameters...
Definition: Colour3DPlotExporter.h:63
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const override
Definition: Colour3DPlotExporter.cpp:121
sv_frame_t getStartFrame() const override
Definition: Colour3DPlotExporter.h:93
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
sv_frame_t getTrueEndFrame() const override
Definition: Colour3DPlotExporter.h:100
Colour3DPlotExporter(Sources sources, Parameters parameters)
Definition: Colour3DPlotExporter.cpp:22
Interface for layers in which the Y axis corresponds to bin number rather than scale value...
Definition: VerticalBinLayer.h:28
QVector< QString > getStringExportHeaders(DataExportOptions options) const override
Definition: Colour3DPlotExporter.cpp:49
double threshold
Threshold below which every value is mapped to background pixel 0 in the display, matching the Colour...
Definition: Colour3DPlotExporter.h:57
int getCompletion() const override
Definition: Colour3DPlotExporter.h:121
~Colour3DPlotExporter()
Definition: Colour3DPlotExporter.cpp:30
const VerticalBinLayer * verticalBinLayer
Definition: Colour3DPlotExporter.h:28
Generated by
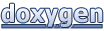