svgui
1.9
|
ScrollableMagRangeCache.h
Go to the documentation of this file.
sv_frame_t getStartFrame() const
Definition: ScrollableMagRangeCache.h:78
MagnitudeRange getRange(int column) const
Get the magnitude range for a single column.
Definition: ScrollableMagRangeCache.h:109
ZoomLevel getZoomLevel() const
Definition: ScrollableMagRangeCache.h:60
bool isColumnSet(int column) const
Definition: ScrollableMagRangeCache.h:95
void resize(int newWidth)
Set the width of the cache in columns.
Definition: ScrollableMagRangeCache.h:54
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
bool areColumnsSet(int x, int count) const
Definition: ScrollableMagRangeCache.h:99
std::vector< MagnitudeRange > m_ranges
Definition: ScrollableMagRangeCache.h:134
ScrollableMagRangeCache()
Definition: ScrollableMagRangeCache.h:38
void sampleColumn(int column, const MagnitudeRange &r)
Update a column in the cache, by column index.
Definition: ScrollableMagRangeCache.cpp:121
sv_frame_t m_startFrame
Definition: ScrollableMagRangeCache.h:135
A cached set of magnitude range records for a view that scrolls horizontally, such as a spectrogram...
Definition: ScrollableMagRangeCache.h:35
void scrollTo(const LayerGeometryProvider *v, sv_frame_t newStartFrame)
Set the new start frame for the cache, according to the geometry of the supplied LayerGeometryProvide...
Definition: ScrollableMagRangeCache.cpp:26
Generated by
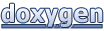