svgui
1.9
|
ScrollableMagRangeCache.cpp
Go to the documentation of this file.
MagnitudeRange getRange(int column) const
Get the magnitude range for a single column.
Definition: ScrollableMagRangeCache.h:109
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
void sampleColumn(int column, const MagnitudeRange &r)
Update a column in the cache, by column index.
Definition: ScrollableMagRangeCache.cpp:121
virtual int getXForFrame(sv_frame_t frame) const =0
Return the pixel x-coordinate corresponding to a given sample frame (which may be negative)...
void scrollTo(const LayerGeometryProvider *v, sv_frame_t newStartFrame)
Set the new start frame for the cache, according to the geometry of the supplied LayerGeometryProvide...
Definition: ScrollableMagRangeCache.cpp:26
Generated by
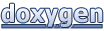