svgui
1.9
|
RangeInputDialog.cpp
Go to the documentation of this file.
void getRange(float &start, float &end)
Definition: RangeInputDialog.cpp:71
void rangeStartChanged(double)
Definition: RangeInputDialog.cpp:99
void rangeChanged(float start, float end)
RangeInputDialog(QString title, QString message, QString unit, float min, float max, QWidget *parent=0)
Definition: RangeInputDialog.cpp:25
Generated by
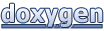