svgui
1.9
|
LinearColourScale.cpp
Go to the documentation of this file.
virtual QColor getColourForValue(LayerGeometryProvider *v, double value) const =0
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
Interface for layers in which a colour scale represents (or can sometimes represent, depending on the display mode) the sample value.
Definition: ColourScaleLayer.h:31
int getWidth(LayerGeometryProvider *v, QPainter &paint)
Definition: LinearColourScale.cpp:26
virtual int getPaintHeight() const
Definition: LayerGeometryProvider.h:188
void paintVertical(LayerGeometryProvider *v, const ColourScaleLayer *layer, QPainter &paint, int x0, double minf, double maxf)
Definition: LinearColourScale.cpp:38
virtual QString getScaleUnits() const =0
Generated by
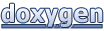