svgui
1.9
|
ItemEditDialog.h
Go to the documentation of this file.
QSpinBox * m_realDurationSecsSpinBox
Definition: ItemEditDialog.h:103
void setFrameDuration(sv_frame_t frame)
Definition: ItemEditDialog.cpp:267
void frameDurationChanged(int)
Definition: ItemEditDialog.cpp:381
void realDurationUSecsChanged(int)
Definition: ItemEditDialog.cpp:406
QSpinBox * m_realTimeUSecsSpinBox
Definition: ItemEditDialog.h:101
Definition: ItemEditDialog.h:34
QSpinBox * m_realTimeSecsSpinBox
Definition: ItemEditDialog.h:100
Definition: ItemEditDialog.h:28
Definition: ItemEditDialog.h:37
void realTimeUSecsChanged(int)
Definition: ItemEditDialog.cpp:371
QSpinBox * m_frameDurationSpinBox
Definition: ItemEditDialog.h:102
void realDurationSecsChanged(int)
Definition: ItemEditDialog.cpp:396
ItemEditDialog(sv_samplerate_t sampleRate, int options, LabelOptions labelOptions={}, QWidget *parent=0)
Definition: ItemEditDialog.cpp:47
Definition: ItemEditDialog.h:36
Definition: ItemEditDialog.h:35
Definition: ItemEditDialog.h:41
QSpinBox * m_realDurationUSecsSpinBox
Definition: ItemEditDialog.h:104
Definition: ItemEditDialog.h:38
sv_frame_t getFrameDuration() const
Definition: ItemEditDialog.cpp:280
Generated by
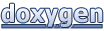