svgui
1.9
|
ColourComboBox.cpp
Go to the documentation of this file.
Very trivial enhancement to QComboBox to make it emit signals when the mouse enters and leaves (for c...
Definition: NotifyingComboBox.h:26
QString getColourName(int c) const
Return the name of the colour at index c.
Definition: ColourDatabase.cpp:43
void includeUnsetEntry(QString label)
Add an entry at the top of the combo for "no colour selected", with the given label.
Definition: ColourComboBox.cpp:44
void colourChanged(int colourIndex)
Emitted when the current index is changed.
void setUseDarkBackground(int c, bool dark)
Mark the colour at index c as using a dark background.
Definition: ColourDatabase.cpp:190
int getColourCount() const
Return the number of colours in the database.
Definition: ColourDatabase.cpp:37
Definition: ColourNameDialog.h:28
Definition: ColourDatabase.h:26
ColourComboBox(bool withAddNewColourEntry, QWidget *parent=0)
Definition: ColourComboBox.cpp:31
int addColour(QColor c, QString name)
Add a colour to the database, with the associated name.
Definition: ColourDatabase.cpp:200
void showDarkBackgroundCheckbox(QString text)
Definition: ColourNameDialog.cpp:68
QPixmap getExamplePixmap(int c, QSize size) const
Generate a swatch pixmap illustrating the colour at index c.
Definition: ColourDatabase.cpp:284
Generated by
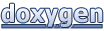