svgui
1.9
|
IconLoader.h
Go to the documentation of this file.
QString makeNonScalableFilename(QString, int, bool)
Definition: IconLoader.cpp:173
Definition: IconLoader.h:21
QString makeScalableFilename(QString, bool)
Definition: IconLoader.cpp:191
Generated by
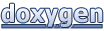