svcore
1.9
|
#include <CSVFileReader.h>
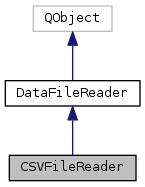
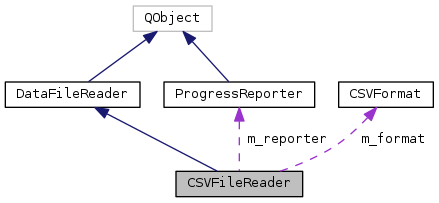
Public Member Functions | |
CSVFileReader (QString path, CSVFormat format, sv_samplerate_t mainModelSampleRate, ProgressReporter *reporter=0) | |
Construct a CSVFileReader to read the CSV file at the given path, with the given format. More... | |
CSVFileReader (QIODevice *device, CSVFormat format, sv_samplerate_t mainModelSampleRate, ProgressReporter *reporter=0) | |
Construct a CSVFileReader to read from the given QIODevice. More... | |
virtual | ~CSVFileReader () |
bool | isOK () const override |
Return true if the file appears to be of the correct type. More... | |
QString | getError () const override |
Model * | load () const override |
Read the file and return the corresponding data model. More... | |
Protected Member Functions | |
bool | convertTimeValue (QString, int lineno, sv_samplerate_t sampleRate, int windowSize, sv_frame_t &calculatedFrame) const |
QString | getConvertedAudioFilePath () const |
Protected Attributes | |
CSVFormat | m_format |
QIODevice * | m_device |
bool | m_ownDevice |
QString | m_filename |
QString | m_error |
int | m_warnings |
sv_samplerate_t | m_mainModelSampleRate |
qint64 | m_fileSize |
qint64 | m_readCount |
int | m_progress |
ProgressReporter * | m_reporter |
Detailed Description
Definition at line 32 of file CSVFileReader.h.
Constructor & Destructor Documentation
CSVFileReader::CSVFileReader | ( | QString | path, |
CSVFormat | format, | ||
sv_samplerate_t | mainModelSampleRate, | ||
ProgressReporter * | reporter = 0 |
||
) |
Construct a CSVFileReader to read the CSV file at the given path, with the given format.
Definition at line 47 of file CSVFileReader.cpp.
References m_device, m_error, m_filename, m_fileSize, m_reporter, and ProgressReporter::setDefinite().
CSVFileReader::CSVFileReader | ( | QIODevice * | device, |
CSVFormat | format, | ||
sv_samplerate_t | mainModelSampleRate, | ||
ProgressReporter * | reporter = 0 |
||
) |
Construct a CSVFileReader to read from the given QIODevice.
Caller retains ownership of the QIODevice: the CSVFileReader will not close or delete it and it must outlive the CSVFileReader.
Definition at line 81 of file CSVFileReader.cpp.
References m_reporter, and ProgressReporter::setDefinite().
|
virtual |
Definition at line 97 of file CSVFileReader.cpp.
References m_device, m_ownDevice, and SVDEBUG.
Member Function Documentation
|
overridevirtual |
Return true if the file appears to be of the correct type.
The DataFileReader will be constructed by passing a file path to its constructor. If the file can at that time be determined to be not of a type that this reader can read, it should return false in response to any subsequent call to isOK().
If the file is apparently of the correct type, isOK() should return true; if it turns out that the file cannot after all be read (because it's corrupted or the detection misfired), then the read() function may return NULL.
Implements DataFileReader.
Definition at line 109 of file CSVFileReader.cpp.
References m_device.
|
overridevirtual |
Reimplemented from DataFileReader.
Definition at line 115 of file CSVFileReader.cpp.
References m_error.
|
overridevirtual |
Read the file and return the corresponding data model.
This function is not expected to be thread-safe or reentrant. This function may be interactive (i.e. it's permitted to pop up dialogs and windows and ask the user to specify any details that can't be automatically extracted from the file).
Return NULL if the file cannot be parsed at all (although it's preferable to return a partial model and warn the user).
Caller owns the returned model and must delete it after use.
Implements DataFileReader.
Definition at line 175 of file CSVFileReader.cpp.
References SparseOneDimensionalModel::add(), BoxModel::add(), RegionModel::add(), SparseTimeValueModel::add(), NoteModel::add(), WritableWaveFileModel::addSamples(), CSVFormat::ColumnDuration, CSVFormat::ColumnEndTime, CSVFormat::ColumnLabel, CSVFormat::ColumnPitch, CSVFormat::ColumnStartTime, CSVFormat::ColumnUnknown, CSVFormat::ColumnValue, RegionModel::containsEvent(), convertTimeValue(), CSVFormat::ExplicitTiming, RegionModel::getAllEvents(), CSVFormat::getAllowQuoting(), CSVFormat::getAudioSampleRange(), CSVFormat::getColumnCount(), CSVFormat::getColumnPurpose(), getConvertedAudioFilePath(), CSVFormat::getHeaderStatus(), CSVFormat::getModelType(), CSVFormat::getSampleRate(), CSVFormat::getSeparator(), CSVFormat::getTimeUnits(), CSVFormat::getTimingType(), CSVFormat::getWindowSize(), CSVFormat::HeaderPresent, CSVFormat::ImplicitTiming, Model::isOK(), m_device, m_filename, m_fileSize, m_format, m_mainModelSampleRate, m_progress, m_readCount, m_reporter, WritableWaveFileModel::None, CSVFormat::OneDimensionalModel, WritableWaveFileModel::Peak, RegionModel::remove(), CSVFormat::SampleRangeOther, CSVFormat::SampleRangeSigned1, CSVFormat::SampleRangeSigned32767, CSVFormat::SampleRangeUnsigned255, EditableDenseThreeDimensionalModel::setColumn(), EditableDenseThreeDimensionalModel::setMaximumLevel(), EditableDenseThreeDimensionalModel::setMinimumLevel(), ProgressReporter::setProgress(), EditableDenseThreeDimensionalModel::setResolution(), NoteModel::setScaleUnits(), EditableDenseThreeDimensionalModel::setStartFrame(), StringBits::split(), SVCERR, SVDEBUG, CSVFormat::ThreeDimensionalModel, CSVFormat::TimeMilliseconds, CSVFormat::TimeSeconds, CSVFormat::TwoDimensionalModel, CSVFormat::TwoDimensionalModelWithDuration, CSVFormat::TwoDimensionalModelWithDurationAndExtent, CSVFormat::TwoDimensionalModelWithDurationAndPitch, WritableWaveFileModel::updateModel(), ProgressReporter::wasCancelled(), CSVFormat::WaveFileModel, and WritableWaveFileModel::writeComplete().
|
protected |
Definition at line 121 of file CSVFileReader.cpp.
References CSVFormat::getTimeUnits(), m_format, m_warnings, StringBits::stringToDoubleLocaleFree(), SVCERR, CSVFormat::TimeMilliseconds, CSVFormat::TimeSeconds, and CSVFormat::TimeWindows.
Referenced by load().
|
protected |
Definition at line 682 of file CSVFileReader.cpp.
References RecordDirectory::getConvertedAudioDirectory(), m_filename, and SVCERR.
Referenced by load().
Member Data Documentation
|
protected |
Definition at line 61 of file CSVFileReader.h.
Referenced by convertTimeValue(), and load().
|
protected |
Definition at line 62 of file CSVFileReader.h.
Referenced by CSVFileReader(), isOK(), load(), and ~CSVFileReader().
|
protected |
Definition at line 63 of file CSVFileReader.h.
Referenced by ~CSVFileReader().
|
protected |
Definition at line 64 of file CSVFileReader.h.
Referenced by CSVFileReader(), getConvertedAudioFilePath(), and load().
|
protected |
Definition at line 65 of file CSVFileReader.h.
Referenced by CSVFileReader(), and getError().
|
mutableprotected |
Definition at line 66 of file CSVFileReader.h.
Referenced by convertTimeValue().
|
protected |
Definition at line 67 of file CSVFileReader.h.
Referenced by load().
|
protected |
Definition at line 68 of file CSVFileReader.h.
Referenced by CSVFileReader(), and load().
|
mutableprotected |
Definition at line 69 of file CSVFileReader.h.
Referenced by load().
|
mutableprotected |
Definition at line 70 of file CSVFileReader.h.
Referenced by load().
|
protected |
Definition at line 71 of file CSVFileReader.h.
Referenced by CSVFileReader(), and load().
The documentation for this class was generated from the following files:
Generated by
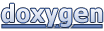