svcore
1.9
|
RecordDirectory.cpp
Go to the documentation of this file.
30 SVCERR << "ERROR: RecordDirectory::getRecordContainerDirectory: Failed to create recorded dir in \"" << parent.canonicalPath() << "\"" << endl;
45 SVCERR << "ERROR: RecordDirectory::getRecordDirectory: Failed to create recorded dir in \"" << parent.canonicalPath() << "\"" << endl;
60 SVCERR << "ERROR: RecordDirectory::getConvertedAudioDirectory: Failed to create recorded dir in \"" << parent.canonicalPath() << "\"" << endl;
static QString getRecordDirectory()
Return the directory in which a recorded file should be saved.
Definition: RecordDirectory.cpp:38
static QString getRecordContainerDirectory()
Return the root "recorded files" directory.
Definition: RecordDirectory.cpp:24
static QString getConvertedAudioDirectory()
Return the directory in which an audio file converted from a data file should be saved.
Definition: RecordDirectory.cpp:53
Generated by
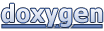