svcore
1.9
|
#include <CSVFormat.h>
Public Types | |
enum | ModelType { OneDimensionalModel, TwoDimensionalModel, TwoDimensionalModelWithDuration, TwoDimensionalModelWithDurationAndPitch, TwoDimensionalModelWithDurationAndExtent, ThreeDimensionalModel, WaveFileModel } |
enum | TimingType { ExplicitTiming, ImplicitTiming } |
enum | TimeUnits { TimeSeconds, TimeMilliseconds, TimeAudioFrames, TimeWindows } |
enum | ColumnPurpose { ColumnUnknown, ColumnStartTime, ColumnEndTime, ColumnDuration, ColumnValue, ColumnPitch, ColumnLabel } |
enum | HeaderStatus { HeaderUnknown = 0, HeaderAbsent = 1, HeaderPresent = 2 } |
enum | ColumnQuality { ColumnNumeric = 1, ColumnIntegral = 2, ColumnIncreasing = 4, ColumnSmall = 8, ColumnLarge = 16, ColumnSigned = 32, ColumnNearEmpty = 64 } |
enum | AudioSampleRange { SampleRangeSigned1 = 0, SampleRangeUnsigned255, SampleRangeSigned32767, SampleRangeOther } |
typedef unsigned int | ColumnQualities |
Protected Member Functions | |
void | guessSeparator (QString line) |
void | guessQualities (QString line, int lineno) |
void | guessPurposes () |
void | guessAudioSampleRange () |
Protected Attributes | |
ModelType | m_modelType |
TimingType | m_timingType |
TimeUnits | m_timeUnits |
QString | m_separator |
std::set< QChar > | m_plausibleSeparators |
sv_samplerate_t | m_sampleRate |
int | m_windowSize |
HeaderStatus | m_headerStatus |
int | m_columnCount |
bool | m_variableColumnCount |
std::map< int, ColumnQualities > | m_columnQualities |
std::map< int, ColumnPurpose > | m_columnPurposes |
std::map< int, QString > | m_columnHeadings |
std::map< int, float > | m_prevValues |
AudioSampleRange | m_audioSampleRange |
bool | m_allowQuoting |
QList< QStringList > | m_example |
int | m_maxExampleCols |
Detailed Description
Definition at line 27 of file CSVFormat.h.
Member Typedef Documentation
typedef unsigned int CSVFormat::ColumnQualities |
Definition at line 77 of file CSVFormat.h.
Member Enumeration Documentation
enum CSVFormat::ModelType |
Enumerator | |
---|---|
OneDimensionalModel | |
TwoDimensionalModel | |
TwoDimensionalModelWithDuration | |
TwoDimensionalModelWithDurationAndPitch | |
TwoDimensionalModelWithDurationAndExtent | |
ThreeDimensionalModel | |
WaveFileModel |
Definition at line 30 of file CSVFormat.h.
Enumerator | |
---|---|
ExplicitTiming | |
ImplicitTiming |
Definition at line 40 of file CSVFormat.h.
enum CSVFormat::TimeUnits |
Enumerator | |
---|---|
TimeSeconds | |
TimeMilliseconds | |
TimeAudioFrames | |
TimeWindows |
Definition at line 45 of file CSVFormat.h.
Enumerator | |
---|---|
ColumnUnknown | |
ColumnStartTime | |
ColumnEndTime | |
ColumnDuration | |
ColumnValue | |
ColumnPitch | |
ColumnLabel |
Definition at line 52 of file CSVFormat.h.
Enumerator | |
---|---|
HeaderUnknown | |
HeaderAbsent | |
HeaderPresent |
Definition at line 62 of file CSVFormat.h.
Enumerator | |
---|---|
ColumnNumeric | |
ColumnIntegral | |
ColumnIncreasing | |
ColumnSmall | |
ColumnLarge | |
ColumnSigned | |
ColumnNearEmpty |
Definition at line 68 of file CSVFormat.h.
Enumerator | |
---|---|
SampleRangeSigned1 | |
SampleRangeUnsigned255 | |
SampleRangeSigned32767 | |
SampleRangeOther |
Definition at line 79 of file CSVFormat.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 86 of file CSVFormat.h.
References guessFormatFor().
CSVFormat::CSVFormat | ( | QString | path | ) |
Definition at line 30 of file CSVFormat.cpp.
References guessFormatFor().
Member Function Documentation
bool CSVFormat::guessFormatFor | ( | QString | path | ) |
Guess the format of the given CSV file, setting the fields in this object accordingly.
If the current separator is the empty string, the separator character will also be guessed; otherwise the current separator will be used. The other properties of this object will be set according to guesses from the file.
The properties that are guessed from the file contents are: separator, column count, variable-column-count flag, audio sample range, timing type, time units, column qualities, column purposes, and model type. The sample rate and window size cannot be guessed and will not be changed by this function. Note also that this function will never guess WaveFileModel for the model type.
Return false if there is some fundamental error, e.g. the file could not be opened at all. Return true otherwise. Note that this function returns true even if the file doesn't appear to make much sense as a data format.
Definition at line 42 of file CSVFormat.cpp.
References ExplicitTiming, guessAudioSampleRange(), guessPurposes(), guessQualities(), m_columnCount, m_columnPurposes, m_columnQualities, m_example, m_maxExampleCols, m_modelType, m_prevValues, m_timeUnits, m_timingType, m_variableColumnCount, SVCERR, SVDEBUG, TimeSeconds, and TwoDimensionalModel.
Referenced by CSVFormat().
|
inline |
Definition at line 125 of file CSVFormat.h.
References m_modelType.
Referenced by CSVFileReader::load().
|
inline |
Definition at line 126 of file CSVFormat.h.
References m_timingType.
Referenced by CSVFileReader::load().
|
inline |
Definition at line 127 of file CSVFormat.h.
References m_timeUnits.
Referenced by CSVFileReader::convertTimeValue(), and CSVFileReader::load().
|
inline |
Definition at line 128 of file CSVFormat.h.
References m_sampleRate.
Referenced by CSVFileReader::load().
|
inline |
Definition at line 129 of file CSVFormat.h.
References m_windowSize.
Referenced by CSVFileReader::load().
|
inline |
Definition at line 130 of file CSVFormat.h.
References m_columnCount.
Referenced by CSVFileReader::load().
|
inline |
Definition at line 131 of file CSVFormat.h.
References m_audioSampleRange.
Referenced by CSVFileReader::load().
|
inline |
Definition at line 132 of file CSVFormat.h.
References m_allowQuoting.
Referenced by CSVFileReader::load().
|
inline |
Definition at line 133 of file CSVFormat.h.
References m_headerStatus.
Referenced by CSVFileReader::load().
|
inline |
Definition at line 134 of file CSVFormat.h.
References m_separator.
Referenced by guessQualities(), and CSVFileReader::load().
|
inline |
Definition at line 139 of file CSVFormat.h.
References m_plausibleSeparators.
|
inline |
Definition at line 143 of file CSVFormat.h.
References m_modelType.
|
inline |
Definition at line 144 of file CSVFormat.h.
References m_timingType.
|
inline |
Definition at line 145 of file CSVFormat.h.
References m_timeUnits.
|
inline |
Definition at line 146 of file CSVFormat.h.
References m_separator.
|
inline |
Definition at line 147 of file CSVFormat.h.
References m_sampleRate.
|
inline |
Definition at line 148 of file CSVFormat.h.
References m_windowSize.
|
inline |
Definition at line 149 of file CSVFormat.h.
References m_columnCount.
|
inline |
Definition at line 150 of file CSVFormat.h.
References m_audioSampleRange.
|
inline |
Definition at line 151 of file CSVFormat.h.
References m_allowQuoting.
|
inline |
Definition at line 152 of file CSVFormat.h.
References getColumnPurpose(), getColumnPurposes(), getColumnQualities(), m_headerStatus, setColumnPurpose(), and setColumnPurposes().
QList< CSVFormat::ColumnPurpose > CSVFormat::getColumnPurposes | ( | ) | const |
Definition at line 535 of file CSVFormat.cpp.
References getColumnPurpose(), and m_columnCount.
Referenced by setHeaderStatus().
void CSVFormat::setColumnPurposes | ( | QList< ColumnPurpose > | cl | ) |
Definition at line 545 of file CSVFormat.cpp.
References in_range_for(), and m_columnPurposes.
Referenced by setHeaderStatus().
CSVFormat::ColumnPurpose CSVFormat::getColumnPurpose | ( | int | i | ) | const |
Definition at line 554 of file CSVFormat.cpp.
References ColumnUnknown, and m_columnPurposes.
Referenced by getColumnPurposes(), CSVFileReader::load(), and setHeaderStatus().
void CSVFormat::setColumnPurpose | ( | int | i, |
ColumnPurpose | p | ||
) |
Definition at line 564 of file CSVFormat.cpp.
References m_columnPurposes.
Referenced by guessPurposes(), and setHeaderStatus().
QList< CSVFormat::ColumnQualities > CSVFormat::getColumnQualities | ( | ) | const |
Definition at line 570 of file CSVFormat.cpp.
References m_columnCount, and m_columnQualities.
Referenced by setHeaderStatus().
|
inline |
Definition at line 164 of file CSVFormat.h.
References m_example.
|
inline |
Definition at line 165 of file CSVFormat.h.
References m_maxExampleCols.
|
protected |
Definition at line 104 of file CSVFormat.cpp.
References m_allowQuoting, m_plausibleSeparators, m_separator, StringBits::split(), and SVDEBUG.
Referenced by guessQualities().
|
protected |
Definition at line 122 of file CSVFormat.cpp.
References ColumnIncreasing, ColumnIntegral, ColumnLarge, ColumnNearEmpty, ColumnNumeric, ColumnSigned, ColumnSmall, getSeparator(), guessSeparator(), HeaderAbsent, HeaderPresent, HeaderUnknown, m_allowQuoting, m_columnCount, m_columnHeadings, m_columnQualities, m_example, m_headerStatus, m_maxExampleCols, m_prevValues, m_variableColumnCount, StringBits::split(), StringBits::stringToDoubleLocaleFree(), and SVDEBUG.
Referenced by guessFormatFor().
|
protected |
Definition at line 292 of file CSVFormat.cpp.
References ColumnDuration, ColumnEndTime, ColumnIncreasing, ColumnIntegral, ColumnLabel, ColumnLarge, ColumnNearEmpty, ColumnNumeric, ColumnStartTime, ColumnUnknown, ColumnValue, ExplicitTiming, ImplicitTiming, m_columnCount, m_columnHeadings, m_columnPurposes, m_columnQualities, m_modelType, m_timeUnits, m_timingType, OneDimensionalModel, setColumnPurpose(), SVDEBUG, ThreeDimensionalModel, TimeAudioFrames, TimeSeconds, TimeWindows, TwoDimensionalModel, and TwoDimensionalModelWithDuration.
Referenced by guessFormatFor().
|
protected |
Definition at line 467 of file CSVFormat.cpp.
References ColumnIntegral, ColumnLarge, ColumnSigned, ColumnSmall, ColumnValue, m_audioSampleRange, m_columnCount, m_columnPurposes, m_columnQualities, SampleRangeOther, SampleRangeSigned1, SampleRangeSigned32767, SampleRangeUnsigned255, and SVDEBUG.
Referenced by guessFormatFor().
Member Data Documentation
|
protected |
Definition at line 168 of file CSVFormat.h.
Referenced by getModelType(), guessFormatFor(), guessPurposes(), and setModelType().
|
protected |
Definition at line 169 of file CSVFormat.h.
Referenced by getTimingType(), guessFormatFor(), guessPurposes(), and setTimingType().
|
protected |
Definition at line 170 of file CSVFormat.h.
Referenced by getTimeUnits(), guessFormatFor(), guessPurposes(), and setTimeUnits().
|
protected |
Definition at line 171 of file CSVFormat.h.
Referenced by getSeparator(), guessSeparator(), and setSeparator().
|
protected |
Definition at line 172 of file CSVFormat.h.
Referenced by getPlausibleSeparators(), and guessSeparator().
|
protected |
Definition at line 173 of file CSVFormat.h.
Referenced by getSampleRate(), and setSampleRate().
|
protected |
Definition at line 174 of file CSVFormat.h.
Referenced by getWindowSize(), and setWindowSize().
|
protected |
Definition at line 175 of file CSVFormat.h.
Referenced by getHeaderStatus(), guessQualities(), and setHeaderStatus().
|
protected |
Definition at line 177 of file CSVFormat.h.
Referenced by getColumnCount(), getColumnPurposes(), getColumnQualities(), guessAudioSampleRange(), guessFormatFor(), guessPurposes(), guessQualities(), and setColumnCount().
|
protected |
Definition at line 178 of file CSVFormat.h.
Referenced by guessFormatFor(), and guessQualities().
|
protected |
Definition at line 180 of file CSVFormat.h.
Referenced by getColumnQualities(), guessAudioSampleRange(), guessFormatFor(), guessPurposes(), and guessQualities().
|
protected |
Definition at line 181 of file CSVFormat.h.
Referenced by getColumnPurpose(), guessAudioSampleRange(), guessFormatFor(), guessPurposes(), setColumnPurpose(), and setColumnPurposes().
|
protected |
Definition at line 182 of file CSVFormat.h.
Referenced by guessPurposes(), and guessQualities().
|
protected |
Definition at line 184 of file CSVFormat.h.
Referenced by guessFormatFor(), and guessQualities().
|
protected |
Definition at line 186 of file CSVFormat.h.
Referenced by getAudioSampleRange(), guessAudioSampleRange(), and setAudioSampleRange().
|
protected |
Definition at line 188 of file CSVFormat.h.
Referenced by getAllowQuoting(), guessQualities(), guessSeparator(), and setAllowQuoting().
|
protected |
Definition at line 190 of file CSVFormat.h.
Referenced by getExample(), guessFormatFor(), and guessQualities().
|
protected |
Definition at line 191 of file CSVFormat.h.
Referenced by getMaxExampleCols(), guessFormatFor(), and guessQualities().
The documentation for this class was generated from the following files:
Generated by
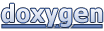