svcore
1.9
|
CSVFormat.cpp
Go to the documentation of this file.
151 SVDEBUG << "line no " << lineno << ": column " << i << " contains: \"" << list[i] << "\"" << endl;
Definition: CSVFormat.h:42
Definition: CSVFormat.h:64
Definition: CSVFormat.h:83
Definition: CSVFormat.h:41
Definition: CSVFormat.h:56
static QStringList split(QString s, QChar separator, bool quoted)
Split a string at the given separator character.
Definition: StringBits.cpp:160
Definition: CSVFormat.h:49
bool guessFormatFor(QString path)
Guess the format of the given CSV file, setting the fields in this object accordingly.
Definition: CSVFormat.cpp:42
QList< ColumnPurpose > getColumnPurposes() const
Definition: CSVFormat.cpp:535
Definition: CSVFormat.h:48
Definition: CSVFormat.h:63
Definition: CSVFormat.h:53
Definition: CSVFormat.h:46
Definition: CSVFormat.h:71
Definition: CSVFormat.h:75
Definition: CSVFormat.h:55
std::map< int, ColumnPurpose > m_columnPurposes
Definition: CSVFormat.h:181
ColumnPurpose getColumnPurpose(int i) const
Definition: CSVFormat.cpp:554
std::map< int, ColumnQualities > m_columnQualities
Definition: CSVFormat.h:180
Definition: CSVFormat.h:31
Definition: CSVFormat.h:59
Definition: CSVFormat.h:70
Definition: CSVFormat.h:57
Definition: CSVFormat.h:74
void setColumnPurpose(int i, ColumnPurpose p)
Definition: CSVFormat.cpp:564
Definition: CSVFormat.h:69
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
Definition: CSVFormat.h:80
Definition: CSVFormat.h:72
Definition: CSVFormat.h:81
void setColumnPurposes(QList< ColumnPurpose > cl)
Definition: CSVFormat.cpp:545
Definition: CSVFormat.h:36
Definition: CSVFormat.h:73
Definition: CSVFormat.h:65
Definition: CSVFormat.h:32
Definition: CSVFormat.h:54
Definition: CSVFormat.h:82
QList< ColumnQualities > getColumnQualities() const
Definition: CSVFormat.cpp:570
static double stringToDoubleLocaleFree(QString s, bool *ok=0)
Convert a string to a double using basic "C"-locale syntax, i.e.
Definition: StringBits.cpp:28
Generated by
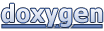