svcore
1.9
|
CSVFileReader.h
Go to the documentation of this file.
Definition: CSVFormat.h:27
Definition: ProgressReporter.h:22
QString getConvertedAudioFilePath() const
Definition: CSVFileReader.cpp:682
Definition: DataFileReader.h:24
CSVFileReader(QString path, CSVFormat format, sv_samplerate_t mainModelSampleRate, ProgressReporter *reporter=0)
Construct a CSVFileReader to read the CSV file at the given path, with the given format.
Definition: CSVFileReader.cpp:47
bool isOK() const override
Return true if the file appears to be of the correct type.
Definition: CSVFileReader.cpp:109
Definition: CSVFileReader.h:32
sv_samplerate_t m_mainModelSampleRate
Definition: CSVFileReader.h:67
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
bool convertTimeValue(QString, int lineno, sv_samplerate_t sampleRate, int windowSize, sv_frame_t &calculatedFrame) const
Definition: CSVFileReader.cpp:121
Model * load() const override
Read the file and return the corresponding data model.
Definition: CSVFileReader.cpp:175
Generated by
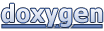