qm-dsp
1.8
|
Static helper functions for simple mathematical calculations. More...
#include <MathUtilities.h>
Public Types | |
enum | NormaliseType { NormaliseNone, NormaliseUnitSum, NormaliseUnitMax } |
Static Public Member Functions | |
static double | round (double x) |
Round x to the nearest integer. More... | |
static void | getFrameMinMax (const double *data, int len, double *min, double *max) |
Return through min and max pointers the highest and lowest values in the given array of the given length. More... | |
static double | mean (const double *src, int len) |
Return the mean of the given array of the given length. More... | |
static double | mean (const std::vector< double > &data, int start, int count) |
Return the mean of the subset of the given vector identified by start and count. More... | |
static double | sum (const double *src, int len) |
Return the sum of the values in the given array of the given length. More... | |
static double | median (const double *src, int len) |
Return the median of the values in the given array of the given length. More... | |
static double | princarg (double ang) |
The principle argument function. More... | |
static double | mod (double x, double y) |
Floating-point division modulus: return x % y. More... | |
static void | getAlphaNorm (const double *data, int len, int alpha, double *ANorm) |
The alpha norm is the alpha'th root of the mean alpha'th power magnitude. More... | |
static double | getAlphaNorm (const std::vector< double > &data, int alpha) |
The alpha norm is the alpha'th root of the mean alpha'th power magnitude. More... | |
static void | normalise (double *data, int length, NormaliseType n=NormaliseUnitMax) |
static void | normalise (std::vector< double > &data, NormaliseType n=NormaliseUnitMax) |
static double | getLpNorm (const std::vector< double > &data, int p) |
Calculate the L^p norm of a vector. More... | |
static std::vector< double > | normaliseLp (const std::vector< double > &data, int p, double threshold=1e-6) |
Normalise a vector by dividing through by its L^p norm. More... | |
static void | adaptiveThreshold (std::vector< double > &data) |
Threshold the input/output vector data against a moving-mean average filter. More... | |
static void | circShift (double *data, int length, int shift) |
static int | getMax (double *data, int length, double *max=0) |
static int | getMax (const std::vector< double > &data, double *max=0) |
static int | compareInt (const void *a, const void *b) |
static bool | isPowerOfTwo (int x) |
Return true if x is 2^n for some integer n >= 0. More... | |
static int | nextPowerOfTwo (int x) |
Return the next higher integer power of two from x, e.g. More... | |
static int | previousPowerOfTwo (int x) |
Return the next lower integer power of two from x, e.g. More... | |
static int | nearestPowerOfTwo (int x) |
Return the nearest integer power of two to x, e.g. More... | |
static double | factorial (int x) |
Return x! More... | |
static int | gcd (int a, int b) |
Return the greatest common divisor of natural numbers a and b. More... | |
Detailed Description
Static helper functions for simple mathematical calculations.
Definition at line 26 of file MathUtilities.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NormaliseNone | |
NormaliseUnitSum | |
NormaliseUnitMax |
Definition at line 93 of file MathUtilities.h.
Member Function Documentation
|
static |
Round x to the nearest integer.
Definition at line 75 of file MathUtilities.cpp.
Referenced by TempoTrack::beatPredict(), TempoTrack::createPhaseExtractor(), TempoTrack::findMeter(), and TempoTrack::phaseMM().
|
static |
Return through min and max pointers the highest and lowest values in the given array of the given length.
Definition at line 140 of file MathUtilities.cpp.
Referenced by TempoTrack::createPhaseExtractor(), DFProcess::removeDCNormalize(), and Chromagram::unityNormalise().
|
static |
Return the mean of the given array of the given length.
Definition at line 112 of file MathUtilities.cpp.
Referenced by GetKeyMode::GetKeyMode(), GetKeyMode::process(), and TempoTrack::tempoMM().
|
static |
Return the mean of the subset of the given vector identified by start and count.
|
static |
Return the sum of the values in the given array of the given length.
Definition at line 100 of file MathUtilities.cpp.
|
static |
Return the median of the values in the given array of the given length.
If the array is even in length, the returned value will be half-way between the two values adjacent to median.
Definition at line 84 of file MathUtilities.cpp.
Referenced by DFProcess::medianFilter().
|
static |
The principle argument function.
Map the phase angle ang into the range [-pi,pi).
Definition at line 33 of file MathUtilities.cpp.
Referenced by DetectionFunction::complexSD(), DetectionFunction::phaseDev(), and PhaseVocoder::unwrapPhases().
|
static |
Floating-point division modulus: return x % y.
Definition at line 25 of file MathUtilities.cpp.
|
static |
The alpha norm is the alpha'th root of the mean alpha'th power magnitude.
For example if alpha = 2 this corresponds to the RMS of the input data, and when alpha = 1 this is the mean magnitude.
Definition at line 42 of file MathUtilities.cpp.
Referenced by DFProcess::removeDCNormalize().
|
static |
The alpha norm is the alpha'th root of the mean alpha'th power magnitude.
For example if alpha = 2 this corresponds to the RMS of the input data, and when alpha = 1 this is the mean magnitude.
|
static |
Definition at line 235 of file MathUtilities.cpp.
Referenced by Chromagram::process().
|
static |
|
static |
Calculate the L^p norm of a vector.
Equivalent to MATLAB's norm(data, p).
Definition at line 305 of file MathUtilities.cpp.
|
static |
Normalise a vector by dividing through by its L^p norm.
If the norm is below the given threshold, the unit vector for that norm is returned. p may be 0, in which case no normalisation happens and the data is returned unchanged.
Definition at line 314 of file MathUtilities.cpp.
|
static |
Threshold the input/output vector data against a moving-mean average filter.
Definition at line 331 of file MathUtilities.cpp.
Referenced by DownBeat::findDownBeats(), and TempoTrackV2::get_rcf().
|
static |
Definition at line 212 of file MathUtilities.cpp.
|
static |
Definition at line 165 of file MathUtilities.cpp.
Referenced by DownBeat::findDownBeats(), and GetKeyMode::process().
|
static |
|
static |
Definition at line 230 of file MathUtilities.cpp.
Referenced by GetKeyMode::process().
|
static |
Return true if x is 2^n for some integer n >= 0.
Definition at line 356 of file MathUtilities.cpp.
Referenced by DecimatorB::initialise().
|
static |
Return the next higher integer power of two from x, e.g.
1300 -> 2048, 2048 -> 2048.
Definition at line 364 of file MathUtilities.cpp.
Referenced by DownBeat::DownBeat(), and ConstantQ::initialise().
|
static |
Return the next lower integer power of two from x, e.g.
1300 -> 1024, 2048 -> 2048.
Definition at line 374 of file MathUtilities.cpp.
|
static |
Return the nearest integer power of two to x, e.g.
1300 -> 1024, 12 -> 16 (not 8; if two are equidistant, the higher is returned).
Definition at line 385 of file MathUtilities.cpp.
|
static |
|
static |
Return the greatest common divisor of natural numbers a and b.
Definition at line 406 of file MathUtilities.cpp.
Referenced by Resampler::initialise().
The documentation for this class was generated from the following files:
Generated by
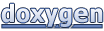