qm-dsp
1.8
|
GetKeyMode.cpp
Go to the documentation of this file.
96 // std::cerr << "chroma frame size = " << m_ChromaFrameSize << ", decimation factor = " << m_DecimationFactor << " therefore block size = " << getBlockSize() << std::endl;
void process(const double *src, double *dst)
Process inLength samples (as supplied to constructor) from src and write inLength / decFactor samples...
Definition: Decimator.cpp:195
Definition: GetKeyMode.h:22
Definition: Chromagram.h:22
double * process(const double *data)
Process a time-domain input signal of length equal to getFrameSize().
Definition: Chromagram.cpp:124
int process(double *pcmData)
Process a single time-domain input sample frame of length getBlockSize().
Definition: GetKeyMode.cpp:192
Definition: Chromagram.h:31
double krumCorr(const double *pDataNorm, const double *pProfileNorm, int shiftProfile, int length)
Definition: GetKeyMode.cpp:161
static int getMax(double *data, int length, double *max=0)
Definition: MathUtilities.cpp:165
double * getKeyStrengths()
Return a pointer to an internal 24-element array containing the correlation of the chroma vector gene...
Definition: GetKeyMode.cpp:292
static float getFrequencyForPitch(int midiPitch, float centsOffset=0, float concertA=440.0)
Definition: Pitch.cpp:20
static int compareInt(const void *a, const void *b)
Definition: MathUtilities.cpp:230
Decimator carries out a fast downsample by a power-of-two factor.
Definition: Decimator.h:24
static double mean(const double *src, int len)
Return the mean of the given array of the given length.
Definition: MathUtilities.cpp:112
Generated by
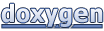