qm-dsp
1.8
|
Decimator.h
Go to the documentation of this file.
void process(const double *src, double *dst)
Process inLength samples (as supplied to constructor) from src and write inLength / decFactor samples...
Definition: Decimator.cpp:195
static int getHighestSupportedFactor()
Definition: Decimator.h:57
Decimator(int inLength, int decFactor)
Construct a Decimator to operate on input blocks of length inLength, with decimation factor decFactor...
Definition: Decimator.cpp:24
void doAntiAlias(const double *src, double *dst, int length)
Definition: Decimator.cpp:155
Decimator carries out a fast downsample by a power-of-two factor.
Definition: Decimator.h:24
Generated by
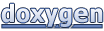