qm-dsp
1.8
|
MathUtilities.h
Go to the documentation of this file.
Static helper functions for simple mathematical calculations.
Definition: MathUtilities.h:26
static void adaptiveThreshold(std::vector< double > &data)
Threshold the input/output vector data against a moving-mean average filter.
Definition: MathUtilities.cpp:331
static void circShift(double *data, int length, int shift)
Definition: MathUtilities.cpp:212
static double mod(double x, double y)
Floating-point division modulus: return x % y.
Definition: MathUtilities.cpp:25
Definition: MathUtilities.h:94
static double sum(const double *src, int len)
Return the sum of the values in the given array of the given length.
Definition: MathUtilities.cpp:100
static int getMax(double *data, int length, double *max=0)
Definition: MathUtilities.cpp:165
static void getFrameMinMax(const double *data, int len, double *min, double *max)
Return through min and max pointers the highest and lowest values in the given array of the given len...
Definition: MathUtilities.cpp:140
static int nextPowerOfTwo(int x)
Return the next higher integer power of two from x, e.g.
Definition: MathUtilities.cpp:364
static int compareInt(const void *a, const void *b)
Definition: MathUtilities.cpp:230
static double getLpNorm(const std::vector< double > &data, int p)
Calculate the L^p norm of a vector.
Definition: MathUtilities.cpp:305
static bool isPowerOfTwo(int x)
Return true if x is 2^n for some integer n >= 0.
Definition: MathUtilities.cpp:356
static int previousPowerOfTwo(int x)
Return the next lower integer power of two from x, e.g.
Definition: MathUtilities.cpp:374
static int gcd(int a, int b)
Return the greatest common divisor of natural numbers a and b.
Definition: MathUtilities.cpp:406
static void getAlphaNorm(const double *data, int len, int alpha, double *ANorm)
The alpha norm is the alpha'th root of the mean alpha'th power magnitude.
Definition: MathUtilities.cpp:42
static int nearestPowerOfTwo(int x)
Return the nearest integer power of two to x, e.g.
Definition: MathUtilities.cpp:385
static double median(const double *src, int len)
Return the median of the values in the given array of the given length.
Definition: MathUtilities.cpp:84
static double mean(const double *src, int len)
Return the mean of the given array of the given length.
Definition: MathUtilities.cpp:112
static void normalise(double *data, int length, NormaliseType n=NormaliseUnitMax)
Definition: MathUtilities.cpp:235
static std::vector< double > normaliseLp(const std::vector< double > &data, int p, double threshold=1e-6)
Normalise a vector by dividing through by its L^p norm.
Definition: MathUtilities.cpp:314
Generated by
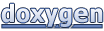