qm-dsp
1.8
|
GetKeyMode.h
Go to the documentation of this file.
Definition: GetKeyMode.h:22
Definition: GetKeyMode.h:19
int process(double *pcmData)
Process a single time-domain input sample frame of length getBlockSize().
Definition: GetKeyMode.cpp:192
Config(double _sampleRate, float _tuningFrequency)
Definition: GetKeyMode.h:32
Definition: Chromagram.h:31
double krumCorr(const double *pDataNorm, const double *pProfileNorm, int shiftProfile, int length)
Definition: GetKeyMode.cpp:161
double * getKeyStrengths()
Return a pointer to an internal 24-element array containing the correlation of the chroma vector gene...
Definition: GetKeyMode.cpp:292
Decimator carries out a fast downsample by a power-of-two factor.
Definition: Decimator.h:24
Generated by
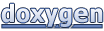