qm-dsp
1.8
|
Pitch.cpp
Go to the documentation of this file.
static float getFrequencyForPitch(int midiPitch, float centsOffset=0, float concertA=440.0)
Definition: Pitch.cpp:20
static int getPitchForFrequency(float frequency, float *centsOffsetReturn=0, float concertA=440.0)
Definition: Pitch.cpp:29
Generated by
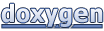