qm-dsp
1.8
|
DFProcess.cpp
Go to the documentation of this file.
Zero-phase digital filter, implemented by processing the data through a filter specified by the given...
Definition: FiltFilt.h:25
Definition: Filter.h:25
static void getFrameMinMax(const double *data, int len, double *min, double *max)
Return through min and max pointers the highest and lowest values in the given array of the given len...
Definition: MathUtilities.cpp:140
void process(const double *const QM_R__ src, double *const QM_R__ dst, const int length)
Definition: FiltFilt.cpp:28
Definition: DFProcess.h:31
static void getAlphaNorm(const double *data, int len, int alpha, double *ANorm)
The alpha norm is the alpha'th root of the mean alpha'th power magnitude.
Definition: MathUtilities.cpp:42
static double median(const double *src, int len)
Return the median of the values in the given array of the given length.
Definition: MathUtilities.cpp:84
void removeDCNormalize(double *src, double *dst)
Definition: DFProcess.cpp:179
Generated by
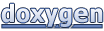