qm-dsp
1.8
|
DFProcess.h
Go to the documentation of this file.
Zero-phase digital filter, implemented by processing the data through a filter specified by the given...
Definition: FiltFilt.h:25
Definition: DFProcess.h:57
Definition: DFProcess.h:31
Generated by
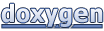