qm-dsp
1.8
|
Filter.h
Go to the documentation of this file.
Filter & operator=(const Filter &)
void process(const double *const QM_R__ in, double *const QM_R__ out, const int n)
Filter the input sequence.
Definition: Filter.cpp:77
Definition: Filter.h:22
Definition: Filter.h:25
Filter(Parameters params)
Construct an IIR filter with numerators b and denominators a.
Definition: Filter.cpp:21
Generated by
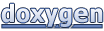