qm-dsp
1.8
|
PhaseVocoder.cpp
Go to the documentation of this file.
void processTimeDomain(const double *src, double *mag, double *phase, double *unwrapped)
Given one frame of time-domain samples, FFT and return the magnitudes, instantaneous phases...
Definition: PhaseVocoder.cpp:66
void forward(const double *realIn, double *realOut, double *imagOut)
Carry out a forward real-to-complex transform of size nsamples, where nsamples is the value provided ...
Definition: FFT.cpp:184
void unwrapPhases(double *theta, double *unwrapped)
Definition: PhaseVocoder.cpp:121
void processFrequencyDomain(const double *reals, const double *imags, double *mag, double *phase, double *unwrapped)
Given one frame of frequency-domain samples, return the magnitudes, instantaneous phases...
Definition: PhaseVocoder.cpp:80
Generated by
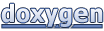