qm-dsp
1.8
|
PhaseVocoder.h
Go to the documentation of this file.
void processTimeDomain(const double *src, double *mag, double *phase, double *unwrapped)
Given one frame of time-domain samples, FFT and return the magnitudes, instantaneous phases...
Definition: PhaseVocoder.cpp:66
Definition: PhaseVocoder.h:21
void unwrapPhases(double *theta, double *unwrapped)
Definition: PhaseVocoder.cpp:121
void processFrequencyDomain(const double *reals, const double *imags, double *mag, double *phase, double *unwrapped)
Given one frame of frequency-domain samples, return the magnitudes, instantaneous phases...
Definition: PhaseVocoder.cpp:80
Generated by
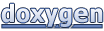