qm-dsp
1.8
|
FFT.h
Go to the documentation of this file.
Definition: FFT.cpp:22
Definition: FFT.cpp:100
void process(bool inverse, const double *realIn, const double *imagIn, double *realOut, double *imagOut)
Carry out a forward or inverse transform (depending on the value of inverse) of size nsamples...
Definition: FFT.cpp:91
FFT(int nsamples)
Construct an FFT object to carry out complex-to-complex transforms of size nsamples.
Definition: FFT.cpp:80
Generated by
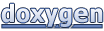