qm-dsp
1.8
|
DetectionFunction.cpp
Go to the documentation of this file.
double processFrequencyDomain(const double *reals, const double *imags)
Process a single frequency-domain frame, provided as frameLength/2+1 real and imaginary component val...
Definition: DetectionFunction.cpp:106
double phaseDev(int length, double *srcPhase)
Definition: DetectionFunction.cpp:196
double broadband(int length, double *srcMagnitude)
Definition: DetectionFunction.cpp:258
double processTimeDomain(const double *samples)
Process a single time-domain frame of audio, provided as frameLength samples.
Definition: DetectionFunction.cpp:94
void processTimeDomain(const double *src, double *mag, double *phase, double *unwrapped)
Given one frame of time-domain samples, FFT and return the magnitudes, instantaneous phases...
Definition: PhaseVocoder.cpp:66
DetectionFunction(DFConfig config)
Definition: DetectionFunction.cpp:23
Definition: Window.h:27
Definition: DetectionFunction.h:30
double complexSD(int length, double *srcMagnitude, double *srcPhase)
Definition: DetectionFunction.cpp:227
Definition: PhaseVocoder.h:21
virtual ~DetectionFunction()
Definition: DetectionFunction.cpp:34
double specDiff(int length, double *src)
Definition: DetectionFunction.cpp:173
void processFrequencyDomain(const double *reals, const double *imags, double *mag, double *phase, double *unwrapped)
Given one frame of frequency-domain samples, return the magnitudes, instantaneous phases...
Definition: PhaseVocoder.cpp:80
double * getSpectrumMagnitude()
Definition: DetectionFunction.cpp:272
Generated by
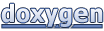