qm-dsp
1.8
|
KaiserWindow.cpp
Go to the documentation of this file.
static Parameters parametersForTransitionWidth(double attenuation, double transition)
Obtain the parameters necessary for a Kaiser window of the given attenuation in dB and transition wid...
Definition: KaiserWindow.cpp:19
Definition: KaiserWindow.h:28
Generated by
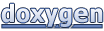