qm-dsp
1.8
|
Kaiser window: A windower whose bandwidth and sidelobe height (signal-noise ratio) can be specified. More...
#include <KaiserWindow.h>
Classes | |
struct | Parameters |
Public Member Functions | |
KaiserWindow (Parameters p) | |
Construct a Kaiser windower with the given length and beta parameter. More... | |
int | getLength () const |
const double * | getWindow () const |
void | cut (double *src) const |
void | cut (const double *src, double *dst) const |
Static Public Member Functions | |
static KaiserWindow | byTransitionWidth (double attenuation, double transition) |
Construct a Kaiser windower with the given attenuation in dB and transition width in samples. More... | |
static KaiserWindow | byBandwidth (double attenuation, double bandwidth, double samplerate) |
Construct a Kaiser windower with the given attenuation in dB and transition bandwidth in Hz for the given samplerate. More... | |
static Parameters | parametersForTransitionWidth (double attenuation, double transition) |
Obtain the parameters necessary for a Kaiser window of the given attenuation in dB and transition width in samples. More... | |
static Parameters | parametersForBandwidth (double attenuation, double bandwidth, double samplerate) |
Obtain the parameters necessary for a Kaiser window of the given attenuation in dB and transition bandwidth in Hz for the given samplerate. More... | |
Private Member Functions | |
void | init () |
Private Attributes | |
int | m_length |
double | m_beta |
std::vector< double > | m_window |
Detailed Description
Kaiser window: A windower whose bandwidth and sidelobe height (signal-noise ratio) can be specified.
These parameters are traded off against the window length.
Definition at line 25 of file KaiserWindow.h.
Constructor & Destructor Documentation
|
inline |
Construct a Kaiser windower with the given length and beta parameter.
Definition at line 37 of file KaiserWindow.h.
References init().
Referenced by byBandwidth(), and byTransitionWidth().
Member Function Documentation
|
inlinestatic |
Construct a Kaiser windower with the given attenuation in dB and transition width in samples.
Definition at line 43 of file KaiserWindow.h.
References KaiserWindow(), and parametersForTransitionWidth().
|
inlinestatic |
Construct a Kaiser windower with the given attenuation in dB and transition bandwidth in Hz for the given samplerate.
Definition at line 53 of file KaiserWindow.h.
References KaiserWindow(), parametersForBandwidth(), and parametersForTransitionWidth().
|
static |
Obtain the parameters necessary for a Kaiser window of the given attenuation in dB and transition width in samples.
Definition at line 19 of file KaiserWindow.cpp.
References KaiserWindow::Parameters::beta, and KaiserWindow::Parameters::length.
Referenced by byBandwidth(), byTransitionWidth(), and parametersForBandwidth().
|
inlinestatic |
Obtain the parameters necessary for a Kaiser window of the given attenuation in dB and transition bandwidth in Hz for the given samplerate.
Definition at line 72 of file KaiserWindow.h.
References parametersForTransitionWidth().
Referenced by byBandwidth(), and Resampler::initialise().
|
inline |
Definition at line 79 of file KaiserWindow.h.
References m_length.
|
inline |
Definition at line 83 of file KaiserWindow.h.
References m_window.
|
inline |
Definition at line 87 of file KaiserWindow.h.
Referenced by Resampler::initialise().
|
inline |
Definition at line 91 of file KaiserWindow.h.
|
private |
Definition at line 54 of file KaiserWindow.cpp.
References bessel0(), m_beta, m_length, and m_window.
Referenced by KaiserWindow().
Member Data Documentation
|
private |
Definition at line 98 of file KaiserWindow.h.
Referenced by cut(), getLength(), and init().
|
private |
Definition at line 99 of file KaiserWindow.h.
Referenced by init().
|
private |
Definition at line 100 of file KaiserWindow.h.
Referenced by cut(), getWindow(), and init().
The documentation for this class was generated from the following files:
Generated by
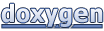