qm-dsp
1.8
|
DownBeat.cpp
Go to the documentation of this file.
148 // DAVIES AND PLUMBLEY "A SPECTRAL DIFFERENCE APPROACH TO EXTRACTING DOWNBEATS IN MUSICAL AUDIO"
void process(const double *src, double *dst)
Process inLength samples (as supplied to constructor) from src and write inLength / decFactor samples...
Definition: Decimator.cpp:195
static void adaptiveThreshold(std::vector< double > &data)
Threshold the input/output vector data against a moving-mean average filter.
Definition: MathUtilities.cpp:331
void getBeatSD(std::vector< double > &beatsd) const
Return the beat spectral difference function.
Definition: DownBeat.cpp:293
void findDownBeats(const float *audio, size_t audioLength, const std::vector< double > &beats, std::vector< int > &downbeats)
Estimate which beats are down-beats.
Definition: DownBeat.cpp:138
static int getHighestSupportedFactor()
Definition: Decimator.h:57
void pushAudioBlock(const float *audio)
For your downsampling convenience: call this function repeatedly with input audio blocks containing d...
Definition: DownBeat.cpp:91
DownBeat(float originalSampleRate, size_t decimationFactor, size_t dfIncrement)
Construct a downbeat locator that will operate on audio at the downsampled by the given decimation fa...
Definition: DownBeat.cpp:28
const float * getBufferedAudio(size_t &length) const
Retrieve the accumulated audio produced by pushAudioBlock calls.
Definition: DownBeat.cpp:120
void forward(const double *realIn, double *realOut, double *imagOut)
Carry out a forward real-to-complex transform of size nsamples, where nsamples is the value provided ...
Definition: FFT.cpp:184
static int getMax(double *data, int length, double *max=0)
Definition: MathUtilities.cpp:165
static int nextPowerOfTwo(int x)
Return the next higher integer power of two from x, e.g.
Definition: MathUtilities.cpp:364
Decimator carries out a fast downsample by a power-of-two factor.
Definition: Decimator.h:24
double measureSpecDiff(d_vec_t oldspec, d_vec_t newspec)
Definition: DownBeat.cpp:245
Generated by
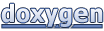