qm-dsp
1.8
|
This class takes an input audio signal and a sequence of beat locations (calculated e.g. More...
#include <DownBeat.h>
Public Member Functions | |
DownBeat (float originalSampleRate, size_t decimationFactor, size_t dfIncrement) | |
Construct a downbeat locator that will operate on audio at the downsampled by the given decimation factor from the given original sample rate, plus beats extracted from the same audio at the given original sample rate with the given frame increment. More... | |
~DownBeat () | |
void | setBeatsPerBar (int bpb) |
void | findDownBeats (const float *audio, size_t audioLength, const std::vector< double > &beats, std::vector< int > &downbeats) |
Estimate which beats are down-beats. More... | |
void | getBeatSD (std::vector< double > &beatsd) const |
Return the beat spectral difference function. More... | |
void | pushAudioBlock (const float *audio) |
For your downsampling convenience: call this function repeatedly with input audio blocks containing dfIncrement samples at the original sample rate, to decimate them to the downsampled rate and buffer them within the DownBeat class. More... | |
const float * | getBufferedAudio (size_t &length) const |
Retrieve the accumulated audio produced by pushAudioBlock calls. More... | |
void | resetAudioBuffer () |
Clear any buffered downsampled audio data. More... | |
Private Types | |
typedef std::vector< int > | i_vec_t |
typedef std::vector< std::vector< int > > | i_mat_t |
typedef std::vector< double > | d_vec_t |
typedef std::vector< std::vector< double > > | d_mat_t |
Private Member Functions | |
void | makeDecimators () |
double | measureSpecDiff (d_vec_t oldspec, d_vec_t newspec) |
Private Attributes | |
int | m_bpb |
float | m_rate |
size_t | m_factor |
size_t | m_increment |
Decimator * | m_decimator1 |
Decimator * | m_decimator2 |
float * | m_buffer |
float * | m_decbuf |
size_t | m_bufsiz |
size_t | m_buffill |
size_t | m_beatframesize |
double * | m_beatframe |
FFTReal * | m_fft |
double * | m_fftRealOut |
double * | m_fftImagOut |
d_vec_t | m_beatsd |
Detailed Description
This class takes an input audio signal and a sequence of beat locations (calculated e.g.
by TempoTrackV2) and estimates which of the beat locations are downbeats (first beat of the bar).
The input audio signal is expected to have been downsampled to a very low sampling rate (e.g. 2700Hz). A utility function for downsampling and buffering incoming block-by-block audio is provided.
Definition at line 36 of file DownBeat.h.
Member Typedef Documentation
|
private |
Definition at line 108 of file DownBeat.h.
|
private |
Definition at line 109 of file DownBeat.h.
|
private |
Definition at line 110 of file DownBeat.h.
|
private |
Definition at line 111 of file DownBeat.h.
Constructor & Destructor Documentation
DownBeat::DownBeat | ( | float | originalSampleRate, |
size_t | decimationFactor, | ||
size_t | dfIncrement | ||
) |
Construct a downbeat locator that will operate on audio at the downsampled by the given decimation factor from the given original sample rate, plus beats extracted from the same audio at the given original sample rate with the given frame increment.
decimationFactor must be a power of two no greater than 64, and dfIncrement must be a multiple of decimationFactor.
Definition at line 28 of file DownBeat.cpp.
References m_beatframe, m_beatframesize, m_fft, m_fftImagOut, m_fftRealOut, m_rate, and MathUtilities::nextPowerOfTwo().
DownBeat::~DownBeat | ( | ) |
Definition at line 58 of file DownBeat.cpp.
References m_beatframe, m_buffer, m_decbuf, m_decimator1, m_decimator2, m_fft, m_fftImagOut, and m_fftRealOut.
Member Function Documentation
void DownBeat::setBeatsPerBar | ( | int | bpb | ) |
Definition at line 71 of file DownBeat.cpp.
References m_bpb.
void DownBeat::findDownBeats | ( | const float * | audio, |
size_t | audioLength, | ||
const std::vector< double > & | beats, | ||
std::vector< int > & | downbeats | ||
) |
Estimate which beats are down-beats.
audio contains the input audio stream after downsampling, and audioLength contains the number of samples in this downsampled stream.
beats contains a series of beat positions expressed in multiples of the df increment at the audio's original sample rate, as described to the constructor.
The returned downbeat array contains a series of indices to the beats array.
Definition at line 138 of file DownBeat.cpp.
References MathUtilities::adaptiveThreshold(), FFTReal::forward(), MathUtilities::getMax(), m_beatframe, m_beatframesize, m_beatsd, m_bpb, m_factor, m_fft, m_fftImagOut, m_fftRealOut, m_increment, measureSpecDiff(), and TWO_PI.
void DownBeat::getBeatSD | ( | std::vector< double > & | beatsd | ) | const |
Return the beat spectral difference function.
This is calculated during findDownBeats, so this function can only be meaningfully called after that has completed. The returned vector contains one value for each of the beat times passed in to findDownBeats, less one. Each value contains the spectral difference between region prior to the beat's nominal position and the region following it.
Definition at line 293 of file DownBeat.cpp.
References m_beatsd.
void DownBeat::pushAudioBlock | ( | const float * | audio | ) |
For your downsampling convenience: call this function repeatedly with input audio blocks containing dfIncrement samples at the original sample rate, to decimate them to the downsampled rate and buffer them within the DownBeat class.
Call getBufferedAudio() to retrieve the results after all blocks have been processed.
Definition at line 91 of file DownBeat.cpp.
References m_buffer, m_buffill, m_bufsiz, m_decbuf, m_decimator1, m_decimator2, m_factor, m_increment, makeDecimators(), and Decimator::process().
const float * DownBeat::getBufferedAudio | ( | size_t & | length | ) | const |
Retrieve the accumulated audio produced by pushAudioBlock calls.
Definition at line 120 of file DownBeat.cpp.
void DownBeat::resetAudioBuffer | ( | ) |
Clear any buffered downsampled audio data.
Definition at line 127 of file DownBeat.cpp.
|
private |
Definition at line 77 of file DownBeat.cpp.
References Decimator::getHighestSupportedFactor(), m_decbuf, m_decimator1, m_decimator2, m_factor, and m_increment.
Referenced by pushAudioBlock().
Member Data Documentation
|
private |
Definition at line 116 of file DownBeat.h.
Referenced by findDownBeats(), and setBeatsPerBar().
|
private |
Definition at line 117 of file DownBeat.h.
Referenced by DownBeat().
|
private |
Definition at line 118 of file DownBeat.h.
Referenced by findDownBeats(), makeDecimators(), and pushAudioBlock().
|
private |
Definition at line 119 of file DownBeat.h.
Referenced by findDownBeats(), makeDecimators(), and pushAudioBlock().
|
private |
Definition at line 120 of file DownBeat.h.
Referenced by makeDecimators(), pushAudioBlock(), and ~DownBeat().
|
private |
Definition at line 121 of file DownBeat.h.
Referenced by makeDecimators(), pushAudioBlock(), and ~DownBeat().
|
private |
Definition at line 122 of file DownBeat.h.
Referenced by getBufferedAudio(), pushAudioBlock(), resetAudioBuffer(), and ~DownBeat().
|
private |
Definition at line 123 of file DownBeat.h.
Referenced by makeDecimators(), pushAudioBlock(), and ~DownBeat().
|
private |
Definition at line 124 of file DownBeat.h.
Referenced by pushAudioBlock(), and resetAudioBuffer().
|
private |
Definition at line 125 of file DownBeat.h.
Referenced by getBufferedAudio(), pushAudioBlock(), and resetAudioBuffer().
|
private |
Definition at line 126 of file DownBeat.h.
Referenced by DownBeat(), and findDownBeats().
|
private |
Definition at line 127 of file DownBeat.h.
Referenced by DownBeat(), findDownBeats(), and ~DownBeat().
|
private |
Definition at line 128 of file DownBeat.h.
Referenced by DownBeat(), findDownBeats(), and ~DownBeat().
|
private |
Definition at line 129 of file DownBeat.h.
Referenced by DownBeat(), findDownBeats(), and ~DownBeat().
|
private |
Definition at line 130 of file DownBeat.h.
Referenced by DownBeat(), findDownBeats(), and ~DownBeat().
|
private |
Definition at line 131 of file DownBeat.h.
Referenced by findDownBeats(), and getBeatSD().
The documentation for this class was generated from the following files:
Generated by
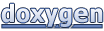