VampPluginSDK
2.10
|
Example plugin that calculates the positions and density of zero-crossing points in an audio waveform. More...
#include <ZeroCrossing.h>
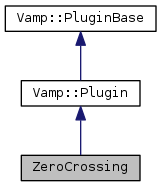
Public Types | |
enum | InputDomain { TimeDomain, FrequencyDomain } |
typedef std::vector< OutputDescriptor > | OutputList |
typedef std::vector< Feature > | FeatureList |
typedef std::map< int, FeatureList > | FeatureSet |
typedef std::vector< ParameterDescriptor > | ParameterList |
typedef std::vector< std::string > | ProgramList |
Public Member Functions | |
ZeroCrossing (float inputSampleRate) | |
virtual | ~ZeroCrossing () |
bool | initialise (size_t channels, size_t stepSize, size_t blockSize) |
Initialise a plugin to prepare it for use with the given number of input channels, step size (window increment, in sample frames) and block size (window size, in sample frames). More... | |
void | reset () |
Reset the plugin after use, to prepare it for another clean run. More... | |
InputDomain | getInputDomain () const |
Get the plugin's required input domain. More... | |
std::string | getIdentifier () const |
Get the computer-usable name of the plugin. More... | |
std::string | getName () const |
Get a human-readable name or title of the plugin. More... | |
std::string | getDescription () const |
Get a human-readable description for the plugin, typically a line of text that may optionally be displayed in addition to the plugin's "name". More... | |
std::string | getMaker () const |
Get the name of the author or vendor of the plugin in human-readable form. More... | |
int | getPluginVersion () const |
Get the version number of the plugin. More... | |
std::string | getCopyright () const |
Get the copyright statement or licensing summary for the plugin. More... | |
OutputList | getOutputDescriptors () const |
Get the outputs of this plugin. More... | |
FeatureSet | process (const float *const *inputBuffers, Vamp::RealTime timestamp) |
Process a single block of input data. More... | |
FeatureSet | getRemainingFeatures () |
After all blocks have been processed, calculate and return any remaining features derived from the complete input. More... | |
virtual size_t | getPreferredBlockSize () const |
Get the preferred block size (window size – the number of sample frames passed in each block to the process() function). More... | |
virtual size_t | getPreferredStepSize () const |
Get the preferred step size (window increment – the distance in sample frames between the start frames of consecutive blocks passed to the process() function) for the plugin. More... | |
virtual size_t | getMinChannelCount () const |
Get the minimum supported number of input channels. More... | |
virtual size_t | getMaxChannelCount () const |
Get the maximum supported number of input channels. More... | |
virtual std::string | getType () const |
Used to distinguish between Vamp::Plugin and other potential sibling subclasses of PluginBase. More... | |
float | getInputSampleRate () const |
Retrieve the input sample rate set on construction. More... | |
virtual unsigned int | getVampApiVersion () const |
Get the Vamp API compatibility level of the plugin. More... | |
virtual ParameterList | getParameterDescriptors () const |
Get the controllable parameters of this plugin. More... | |
virtual float | getParameter (std::string) const |
Get the value of a named parameter. More... | |
virtual void | setParameter (std::string, float) |
Set a named parameter. More... | |
virtual ProgramList | getPrograms () const |
Get the program settings available in this plugin. More... | |
virtual std::string | getCurrentProgram () const |
Get the current program. More... | |
virtual void | selectProgram (std::string) |
Select a program. More... | |
Protected Attributes | |
size_t | m_stepSize |
float | m_previousSample |
float | m_inputSampleRate |
Detailed Description
Example plugin that calculates the positions and density of zero-crossing points in an audio waveform.
Definition at line 47 of file ZeroCrossing.h.
Member Typedef Documentation
|
inherited |
Definition at line 335 of file vamp-sdk/Plugin.h.
|
inherited |
Definition at line 393 of file vamp-sdk/Plugin.h.
|
inherited |
Definition at line 395 of file vamp-sdk/Plugin.h.
|
inherited |
Definition at line 203 of file vamp-sdk/PluginBase.h.
|
inherited |
Definition at line 225 of file vamp-sdk/PluginBase.h.
Member Enumeration Documentation
|
inherited |
Enumerator | |
---|---|
TimeDomain | |
FrequencyDomain |
Definition at line 152 of file vamp-sdk/Plugin.h.
Constructor & Destructor Documentation
ZeroCrossing::ZeroCrossing | ( | float | inputSampleRate | ) |
Definition at line 47 of file ZeroCrossing.cpp.
|
virtual |
Definition at line 54 of file ZeroCrossing.cpp.
Member Function Documentation
|
virtual |
Initialise a plugin to prepare it for use with the given number of input channels, step size (window increment, in sample frames) and block size (window size, in sample frames).
The input sample rate should have been already specified at construction time.
Return true for successful initialisation, false if the number of input channels, step size and/or block size cannot be supported.
Implements Vamp::Plugin.
Definition at line 95 of file ZeroCrossing.cpp.
References Vamp::Plugin::getMaxChannelCount(), Vamp::Plugin::getMinChannelCount(), and m_stepSize.
|
virtual |
Reset the plugin after use, to prepare it for another clean run.
Not called for the first initialisation (i.e. initialise must also do a reset).
Implements Vamp::Plugin.
Definition at line 106 of file ZeroCrossing.cpp.
References m_previousSample.
|
inlinevirtual |
Get the plugin's required input domain.
If this is TimeDomain, the samples provided to the process() function (below) will be in the time domain, as for a traditional audio processing plugin.
If this is FrequencyDomain, the host will carry out a windowed FFT of size equal to the negotiated block size on the data before passing the frequency bin data in to process(). The input data for the FFT will be rotated so as to place the origin in the centre of the block. The plugin does not get to choose the window type – the host will either let the user do so, or will use a Hanning window.
Implements Vamp::Plugin.
Definition at line 56 of file ZeroCrossing.h.
References getCopyright(), getDescription(), getIdentifier(), getMaker(), getName(), getOutputDescriptors(), getPluginVersion(), getRemainingFeatures(), process(), and Vamp::Plugin::TimeDomain.
|
virtual |
Get the computer-usable name of the plugin.
This should be reasonably short and contain no whitespace or punctuation characters. It may only contain the characters [a-zA-Z0-9_-]. This is the authoritative way for a program to identify a plugin within a given library.
This text may be visible to the user, but it should not be the main text used to identify a plugin to the user (that will be the name, below).
Example: "zero_crossings"
Implements Vamp::PluginBase.
Definition at line 59 of file ZeroCrossing.cpp.
Referenced by getInputDomain().
|
virtual |
Get a human-readable name or title of the plugin.
This should be brief and self-contained, as it may be used to identify the plugin to the user in isolation (i.e. without also showing the plugin's "identifier").
Example: "Zero Crossings"
Implements Vamp::PluginBase.
Definition at line 65 of file ZeroCrossing.cpp.
Referenced by getInputDomain().
|
virtual |
Get a human-readable description for the plugin, typically a line of text that may optionally be displayed in addition to the plugin's "name".
May be empty if the name has said it all already.
Example: "Detect and count zero crossing points"
Implements Vamp::PluginBase.
Definition at line 71 of file ZeroCrossing.cpp.
Referenced by getInputDomain().
|
virtual |
Get the name of the author or vendor of the plugin in human-readable form.
This should be a short identifying text, as it may be used to label plugins from the same source in a menu or similar.
Implements Vamp::PluginBase.
Definition at line 77 of file ZeroCrossing.cpp.
Referenced by getInputDomain().
|
virtual |
Get the version number of the plugin.
Implements Vamp::PluginBase.
Definition at line 83 of file ZeroCrossing.cpp.
Referenced by getInputDomain().
|
virtual |
Get the copyright statement or licensing summary for the plugin.
This can be an informative text, without the same presentation constraints as mentioned for getMaker above.
Implements Vamp::PluginBase.
Definition at line 89 of file ZeroCrossing.cpp.
Referenced by getInputDomain().
|
virtual |
Get the outputs of this plugin.
An output's index in this list is used as its numeric index when looking it up in the FeatureSet returned from the process() call.
Implements Vamp::Plugin.
Definition at line 112 of file ZeroCrossing.cpp.
References Vamp::Plugin::OutputDescriptor::binCount, Vamp::Plugin::OutputDescriptor::description, Vamp::Plugin::OutputDescriptor::hasFixedBinCount, Vamp::Plugin::OutputDescriptor::hasKnownExtents, Vamp::Plugin::OutputDescriptor::identifier, Vamp::Plugin::OutputDescriptor::isQuantized, Vamp::Plugin::m_inputSampleRate, Vamp::Plugin::OutputDescriptor::name, Vamp::Plugin::OutputDescriptor::OneSamplePerStep, Vamp::Plugin::OutputDescriptor::quantizeStep, Vamp::Plugin::OutputDescriptor::sampleRate, Vamp::Plugin::OutputDescriptor::sampleType, Vamp::Plugin::OutputDescriptor::unit, and Vamp::Plugin::OutputDescriptor::VariableSampleRate.
Referenced by getInputDomain().
|
virtual |
Process a single block of input data.
If the plugin's inputDomain is TimeDomain, inputBuffers will point to one array of floats per input channel, and each of these arrays will contain blockSize consecutive audio samples (the host will zero-pad as necessary). The timestamp in this case will be the real time in seconds of the start of the supplied block of samples.
If the plugin's inputDomain is FrequencyDomain, inputBuffers will point to one array of floats per input channel, and each of these arrays will contain blockSize/2+1 consecutive pairs of real and imaginary component floats corresponding to bins 0..(blockSize/2) of the FFT output. That is, bin 0 (the first pair of floats) contains the DC output, up to bin blockSize/2 which contains the Nyquist-frequency output. There will therefore be blockSize+2 floats per channel in total. The timestamp will be the real time in seconds of the centre of the FFT input window (i.e. the very first block passed to process might contain the FFT of half a block of zero samples and the first half-block of the actual data, with a timestamp of zero).
Return any features that have become available after this process call. (These do not necessarily have to fall within the process block, except for OneSamplePerStep outputs.)
Implements Vamp::Plugin.
Definition at line 143 of file ZeroCrossing.cpp.
References Vamp::RealTime::frame2RealTime(), Vamp::Plugin::Feature::hasTimestamp, Vamp::Plugin::m_inputSampleRate, m_previousSample, m_stepSize, Vamp::Plugin::Feature::timestamp, and Vamp::Plugin::Feature::values.
Referenced by getInputDomain().
|
virtual |
After all blocks have been processed, calculate and return any remaining features derived from the complete input.
Implements Vamp::Plugin.
Definition at line 192 of file ZeroCrossing.cpp.
Referenced by getInputDomain().
|
inlinevirtualinherited |
Get the preferred block size (window size – the number of sample frames passed in each block to the process() function).
This should be called before initialise().
A plugin that can handle any block size may return 0. The final block size will be set in the initialise() call.
Reimplemented in Vamp::HostExt::PluginBufferingAdapter, Vamp::HostExt::PluginInputDomainAdapter, Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, FixedTempoEstimator, and PercussionOnsetDetector.
Definition at line 179 of file vamp-sdk/Plugin.h.
Referenced by enumeratePlugins(), and runPlugin().
|
inlinevirtualinherited |
Get the preferred step size (window increment – the distance in sample frames between the start frames of consecutive blocks passed to the process() function) for the plugin.
This should be called before initialise().
A plugin may return 0 if it has no particular interest in the step size. In this case, the host should make the step size equal to the block size if the plugin is accepting input in the time domain. If the plugin is accepting input in the frequency domain, the host may use any step size. The final step size will be set in the initialise() call.
Reimplemented in Vamp::HostExt::PluginInputDomainAdapter, Vamp::PluginHostAdapter, Vamp::HostExt::PluginBufferingAdapter, Vamp::HostExt::PluginWrapper, FixedTempoEstimator, and PercussionOnsetDetector.
Definition at line 194 of file vamp-sdk/Plugin.h.
Referenced by enumeratePlugins(), and runPlugin().
|
inlinevirtualinherited |
Get the minimum supported number of input channels.
Reimplemented in Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 199 of file vamp-sdk/Plugin.h.
Referenced by enumeratePlugins(), FixedTempoEstimator::initialise(), PercussionOnsetDetector::initialise(), AmplitudeFollower::initialise(), SpectralCentroid::initialise(), initialise(), PowerSpectrum::initialise(), and runPlugin().
|
inlinevirtualinherited |
Get the maximum supported number of input channels.
Reimplemented in Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 204 of file vamp-sdk/Plugin.h.
Referenced by enumeratePlugins(), FixedTempoEstimator::initialise(), PercussionOnsetDetector::initialise(), AmplitudeFollower::initialise(), SpectralCentroid::initialise(), initialise(), PowerSpectrum::initialise(), and runPlugin().
|
inlinevirtualinherited |
Used to distinguish between Vamp::Plugin and other potential sibling subclasses of PluginBase.
Do not reimplement this function in your subclass.
Implements Vamp::PluginBase.
Definition at line 438 of file vamp-sdk/Plugin.h.
|
inlineinherited |
Retrieve the input sample rate set on construction.
Definition at line 443 of file vamp-sdk/Plugin.h.
|
inlinevirtualinherited |
Get the Vamp API compatibility level of the plugin.
Reimplemented in Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 68 of file vamp-sdk/PluginBase.h.
Referenced by enumeratePlugins().
|
inlinevirtualinherited |
Get the controllable parameters of this plugin.
Reimplemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, AmplitudeFollower, FixedTempoEstimator, and PercussionOnsetDetector.
Definition at line 208 of file vamp-sdk/PluginBase.h.
Referenced by enumeratePlugins().
|
inlinevirtualinherited |
Get the value of a named parameter.
The argument is the identifier field from that parameter's descriptor.
Reimplemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, AmplitudeFollower, FixedTempoEstimator, and PercussionOnsetDetector.
Definition at line 216 of file vamp-sdk/PluginBase.h.
|
inlinevirtualinherited |
Set a named parameter.
The first argument is the identifier field from that parameter's descriptor.
Reimplemented in Vamp::HostExt::PluginBufferingAdapter, Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, AmplitudeFollower, FixedTempoEstimator, and PercussionOnsetDetector.
Definition at line 222 of file vamp-sdk/PluginBase.h.
|
inlinevirtualinherited |
Get the program settings available in this plugin.
A program is a named shorthand for a set of parameter values; changing the program may cause the plugin to alter the values of its published parameters (and/or non-public internal processing parameters). The host should re-read the plugin's parameter values after setting a new program.
The programs must have unique names.
Reimplemented in Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 237 of file vamp-sdk/PluginBase.h.
|
inlinevirtualinherited |
Get the current program.
Reimplemented in Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 242 of file vamp-sdk/PluginBase.h.
|
inlinevirtualinherited |
Select a program.
(If the given program name is not one of the available programs, do nothing.)
Reimplemented in Vamp::HostExt::PluginBufferingAdapter, Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 248 of file vamp-sdk/PluginBase.h.
References _VAMP_SDK_PLUGSPACE_END.
Member Data Documentation
|
protected |
Definition at line 73 of file ZeroCrossing.h.
Referenced by initialise(), and process().
|
protected |
Definition at line 74 of file ZeroCrossing.h.
|
protectedinherited |
Definition at line 449 of file vamp-sdk/Plugin.h.
Referenced by getOutputDescriptors(), PercussionOnsetDetector::getOutputDescriptors(), AmplitudeFollower::initialise(), SpectralCentroid::process(), process(), and PercussionOnsetDetector::process().
The documentation for this class was generated from the following files:
Generated by
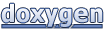