VampPluginSDK
2.10
|
PluginInputDomainAdapter is a Vamp plugin adapter that converts time-domain input into frequency-domain input for plugins that need it. More...
#include <vamp-hostsdk/PluginInputDomainAdapter.h>
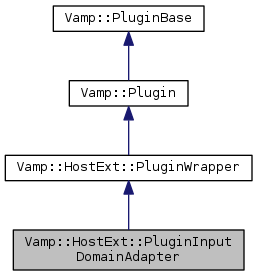
Public Types | |
enum | ProcessTimestampMethod { ShiftTimestamp, ShiftData, NoShift } |
ProcessTimestampMethod determines how the PluginInputDomainAdapter handles timestamps for the data passed to the process() function of the plugin it wraps, in the case where the plugin is expecting frequency-domain data. More... | |
enum | WindowType { RectangularWindow = 0, BartlettWindow = 1, TriangularWindow = 1, HammingWindow = 2, HanningWindow = 3, HannWindow = 3, BlackmanWindow = 4, NuttallWindow = 7, BlackmanHarrisWindow = 8 } |
The set of supported window shapes. More... | |
enum | InputDomain { TimeDomain, FrequencyDomain } |
typedef std::vector< OutputDescriptor > | OutputList |
typedef std::vector< Feature > | FeatureList |
typedef std::map< int, FeatureList > | FeatureSet |
typedef std::vector< ParameterDescriptor > | ParameterList |
typedef std::vector< std::string > | ProgramList |
Public Member Functions | |
PluginInputDomainAdapter (Plugin *plugin) | |
Construct a PluginInputDomainAdapter wrapping the given plugin. More... | |
virtual | ~PluginInputDomainAdapter () |
bool | initialise (size_t channels, size_t stepSize, size_t blockSize) |
Initialise a plugin to prepare it for use with the given number of input channels, step size (window increment, in sample frames) and block size (window size, in sample frames). More... | |
void | reset () |
Reset the plugin after use, to prepare it for another clean run. More... | |
InputDomain | getInputDomain () const |
Get the plugin's required input domain. More... | |
size_t | getPreferredStepSize () const |
Get the preferred step size (window increment – the distance in sample frames between the start frames of consecutive blocks passed to the process() function) for the plugin. More... | |
size_t | getPreferredBlockSize () const |
Get the preferred block size (window size – the number of sample frames passed in each block to the process() function). More... | |
FeatureSet | process (const float *const *inputBuffers, RealTime timestamp) |
Process a single block of input data. More... | |
void | setProcessTimestampMethod (ProcessTimestampMethod) |
Set the method used for timestamp adjustment in plugins taking frequency-domain input. More... | |
ProcessTimestampMethod | getProcessTimestampMethod () const |
Retrieve the method used for timestamp adjustment in plugins taking frequency-domain input. More... | |
RealTime | getTimestampAdjustment () const |
Return the amount by which the timestamps supplied to process() are being incremented when they are passed to the plugin's own process() implementation. More... | |
WindowType | getWindowType () const |
Return the current window shape. More... | |
void | setWindowType (WindowType type) |
Set the current window shape. More... | |
unsigned int | getVampApiVersion () const |
Get the Vamp API compatibility level of the plugin. More... | |
std::string | getIdentifier () const |
Get the computer-usable name of the plugin. More... | |
std::string | getName () const |
Get a human-readable name or title of the plugin. More... | |
std::string | getDescription () const |
Get a human-readable description for the plugin, typically a line of text that may optionally be displayed in addition to the plugin's "name". More... | |
std::string | getMaker () const |
Get the name of the author or vendor of the plugin in human-readable form. More... | |
int | getPluginVersion () const |
Get the version number of the plugin. More... | |
std::string | getCopyright () const |
Get the copyright statement or licensing summary for the plugin. More... | |
ParameterList | getParameterDescriptors () const |
Get the controllable parameters of this plugin. More... | |
float | getParameter (std::string) const |
Get the value of a named parameter. More... | |
void | setParameter (std::string, float) |
Set a named parameter. More... | |
ProgramList | getPrograms () const |
Get the program settings available in this plugin. More... | |
std::string | getCurrentProgram () const |
Get the current program. More... | |
void | selectProgram (std::string) |
Select a program. More... | |
size_t | getMinChannelCount () const |
Get the minimum supported number of input channels. More... | |
size_t | getMaxChannelCount () const |
Get the maximum supported number of input channels. More... | |
OutputList | getOutputDescriptors () const |
Get the outputs of this plugin. More... | |
FeatureSet | getRemainingFeatures () |
After all blocks have been processed, calculate and return any remaining features derived from the complete input. More... | |
template<typename WrapperType > | |
WrapperType * | getWrapper () |
Return a pointer to the plugin wrapper of type WrapperType surrounding this wrapper's plugin, if present. More... | |
void | disownPlugin () |
Disown the wrapped plugin, so that we no longer delete it on our own destruction. More... | |
virtual std::string | getType () const |
Used to distinguish between Vamp::Plugin and other potential sibling subclasses of PluginBase. More... | |
float | getInputSampleRate () const |
Retrieve the input sample rate set on construction. More... | |
Protected Attributes | |
Impl * | m_impl |
Plugin * | m_plugin |
bool | m_pluginIsOwned |
float | m_inputSampleRate |
Detailed Description
PluginInputDomainAdapter is a Vamp plugin adapter that converts time-domain input into frequency-domain input for plugins that need it.
This permits a host to use time- and frequency-domain plugins interchangeably without needing to handle the conversion itself.
This adapter uses a basic windowed FFT (using Hann window by default) that supports power-of-two block sizes only. If a frequency domain plugin requests a non-power-of-two blocksize, the adapter will adjust it to a nearby power of two instead. Thus, getPreferredBlockSize() will always return a power of two if the wrapped plugin is a frequency domain one. If the plugin doesn't accept the adjusted power of two block size, initialise() will fail.
The adapter provides no way for the host to discover whether the underlying plugin is actually a time or frequency domain plugin (except that if the preferred block size is not a power of two, it must be a time domain plugin).
The FFT implementation is simple and self-contained, but unlikely to be the fastest available: a host can usually do better if it cares enough.
The window shape for the FFT frame can be set using setWindowType and the current shape retrieved using getWindowType. (This was added in v2.3 of the SDK.)
In every respect other than its input domain handling, the PluginInputDomainAdapter behaves identically to the plugin that it wraps. The wrapped plugin will be deleted when the wrapper is deleted. If you wish to prevent this, call disownPlugin().
- Note
- This class was introduced in version 1.1 of the Vamp plugin SDK.
Definition at line 87 of file PluginInputDomainAdapter.h.
Member Typedef Documentation
|
inherited |
Definition at line 335 of file vamp-sdk/Plugin.h.
|
inherited |
Definition at line 393 of file vamp-sdk/Plugin.h.
|
inherited |
Definition at line 395 of file vamp-sdk/Plugin.h.
|
inherited |
Definition at line 203 of file vamp-sdk/PluginBase.h.
|
inherited |
Definition at line 225 of file vamp-sdk/PluginBase.h.
Member Enumeration Documentation
ProcessTimestampMethod determines how the PluginInputDomainAdapter handles timestamps for the data passed to the process() function of the plugin it wraps, in the case where the plugin is expecting frequency-domain data.
The Vamp specification requires that the timestamp passed to the plugin for frequency-domain input should be that of the centre of the processing block, rather than the start as is the case for time-domain input.
Since PluginInputDomainAdapter aims to be transparent in use, it needs to handle this timestamp adjustment itself. However, some control is available over the method used for adjustment, by means of the ProcessTimestampMethod setting.
If ProcessTimestampMethod is set to ShiftTimestamp (the default), then the data passed to the wrapped plugin will be calculated from the same input data block as passed to the wrapper, but the timestamp passed to the plugin will be advanced by half of the window size.
If ProcessTimestampMethod is set to ShiftData, then the timestamp passed to the wrapped plugin will be the same as that passed to the process call of the wrapper, but the data block used to calculate the input will be shifted back (earlier) by half of the window size, with half a block of zero padding at the start of the first process call. This has the advantage of preserving the first half block of audio without any deterioration from window shaping.
If ProcessTimestampMethod is set to NoShift, then no adjustment will be made and the timestamps will be incorrect.
Enumerator | |
---|---|
ShiftTimestamp | |
ShiftData | |
NoShift |
Definition at line 143 of file PluginInputDomainAdapter.h.
The set of supported window shapes.
Definition at line 196 of file PluginInputDomainAdapter.h.
|
inherited |
Enumerator | |
---|---|
TimeDomain | |
FrequencyDomain |
Definition at line 152 of file vamp-sdk/Plugin.h.
Constructor & Destructor Documentation
Vamp::HostExt::PluginInputDomainAdapter::PluginInputDomainAdapter | ( | Plugin * | plugin | ) |
Construct a PluginInputDomainAdapter wrapping the given plugin.
The adapter takes ownership of the plugin, which will be deleted when the adapter is deleted. If you wish to prevent this, call disownPlugin().
|
virtual |
Member Function Documentation
|
virtual |
Initialise a plugin to prepare it for use with the given number of input channels, step size (window increment, in sample frames) and block size (window size, in sample frames).
The input sample rate should have been already specified at construction time.
Return true for successful initialisation, false if the number of input channels, step size and/or block size cannot be supported.
Implements Vamp::Plugin.
|
virtual |
Reset the plugin after use, to prepare it for another clean run.
Not called for the first initialisation (i.e. initialise must also do a reset).
Implements Vamp::Plugin.
|
virtual |
Get the plugin's required input domain.
If this is TimeDomain, the samples provided to the process() function (below) will be in the time domain, as for a traditional audio processing plugin.
If this is FrequencyDomain, the host will carry out a windowed FFT of size equal to the negotiated block size on the data before passing the frequency bin data in to process(). The input data for the FFT will be rotated so as to place the origin in the centre of the block. The plugin does not get to choose the window type – the host will either let the user do so, or will use a Hanning window.
Implements Vamp::Plugin.
|
virtual |
Get the preferred step size (window increment – the distance in sample frames between the start frames of consecutive blocks passed to the process() function) for the plugin.
This should be called before initialise().
A plugin may return 0 if it has no particular interest in the step size. In this case, the host should make the step size equal to the block size if the plugin is accepting input in the time domain. If the plugin is accepting input in the frequency domain, the host may use any step size. The final step size will be set in the initialise() call.
Reimplemented from Vamp::Plugin.
|
virtual |
Get the preferred block size (window size – the number of sample frames passed in each block to the process() function).
This should be called before initialise().
A plugin that can handle any block size may return 0. The final block size will be set in the initialise() call.
Reimplemented from Vamp::Plugin.
|
virtual |
Process a single block of input data.
If the plugin's inputDomain is TimeDomain, inputBuffers will point to one array of floats per input channel, and each of these arrays will contain blockSize consecutive audio samples (the host will zero-pad as necessary). The timestamp in this case will be the real time in seconds of the start of the supplied block of samples.
If the plugin's inputDomain is FrequencyDomain, inputBuffers will point to one array of floats per input channel, and each of these arrays will contain blockSize/2+1 consecutive pairs of real and imaginary component floats corresponding to bins 0..(blockSize/2) of the FFT output. That is, bin 0 (the first pair of floats) contains the DC output, up to bin blockSize/2 which contains the Nyquist-frequency output. There will therefore be blockSize+2 floats per channel in total. The timestamp will be the real time in seconds of the centre of the FFT input window (i.e. the very first block passed to process might contain the FFT of half a block of zero samples and the first half-block of the actual data, with a timestamp of zero).
Return any features that have become available after this process call. (These do not necessarily have to fall within the process block, except for OneSamplePerStep outputs.)
Implements Vamp::Plugin.
void Vamp::HostExt::PluginInputDomainAdapter::setProcessTimestampMethod | ( | ProcessTimestampMethod | ) |
Set the method used for timestamp adjustment in plugins taking frequency-domain input.
See the ProcessTimestampMethod documentation for details.
This function must be called before the first call to process().
ProcessTimestampMethod Vamp::HostExt::PluginInputDomainAdapter::getProcessTimestampMethod | ( | ) | const |
Retrieve the method used for timestamp adjustment in plugins taking frequency-domain input.
See the ProcessTimestampMethod documentation for details.
RealTime Vamp::HostExt::PluginInputDomainAdapter::getTimestampAdjustment | ( | ) | const |
Return the amount by which the timestamps supplied to process() are being incremented when they are passed to the plugin's own process() implementation.
The Vamp API mandates that the timestamp passed to the plugin for time-domain input should be the time of the first sample in the block, but the timestamp passed for frequency-domain input should be the timestamp of the centre of the block.
The PluginInputDomainAdapter adjusts its timestamps properly so that the plugin receives correct times, but in some circumstances (such as for establishing the correct timing of implicitly-timed features, i.e. features without their own timestamps) the host may need to be aware that this adjustment is taking place.
If the plugin requires time-domain input or the PluginInputDomainAdapter is configured with its ProcessTimestampMethod set to ShiftData instead of ShiftTimestamp, then this function will return zero.
The result of calling this function before initialise() has been called is undefined.
Referenced by runPlugin().
WindowType Vamp::HostExt::PluginInputDomainAdapter::getWindowType | ( | ) | const |
Return the current window shape.
The default is HanningWindow.
void Vamp::HostExt::PluginInputDomainAdapter::setWindowType | ( | WindowType | type | ) |
Set the current window shape.
|
virtualinherited |
Get the Vamp API compatibility level of the plugin.
Reimplemented from Vamp::PluginBase.
|
virtualinherited |
Get the computer-usable name of the plugin.
This should be reasonably short and contain no whitespace or punctuation characters. It may only contain the characters [a-zA-Z0-9_-]. This is the authoritative way for a program to identify a plugin within a given library.
This text may be visible to the user, but it should not be the main text used to identify a plugin to the user (that will be the name, below).
Example: "zero_crossings"
Implements Vamp::PluginBase.
|
virtualinherited |
Get a human-readable name or title of the plugin.
This should be brief and self-contained, as it may be used to identify the plugin to the user in isolation (i.e. without also showing the plugin's "identifier").
Example: "Zero Crossings"
Implements Vamp::PluginBase.
|
virtualinherited |
Get a human-readable description for the plugin, typically a line of text that may optionally be displayed in addition to the plugin's "name".
May be empty if the name has said it all already.
Example: "Detect and count zero crossing points"
Implements Vamp::PluginBase.
|
virtualinherited |
Get the name of the author or vendor of the plugin in human-readable form.
This should be a short identifying text, as it may be used to label plugins from the same source in a menu or similar.
Implements Vamp::PluginBase.
|
virtualinherited |
Get the version number of the plugin.
Implements Vamp::PluginBase.
|
virtualinherited |
Get the copyright statement or licensing summary for the plugin.
This can be an informative text, without the same presentation constraints as mentioned for getMaker above.
Implements Vamp::PluginBase.
|
virtualinherited |
Get the controllable parameters of this plugin.
Reimplemented from Vamp::PluginBase.
|
virtualinherited |
Get the value of a named parameter.
The argument is the identifier field from that parameter's descriptor.
Reimplemented from Vamp::PluginBase.
|
virtualinherited |
Set a named parameter.
The first argument is the identifier field from that parameter's descriptor.
Reimplemented from Vamp::PluginBase.
|
virtualinherited |
Get the program settings available in this plugin.
A program is a named shorthand for a set of parameter values; changing the program may cause the plugin to alter the values of its published parameters (and/or non-public internal processing parameters). The host should re-read the plugin's parameter values after setting a new program.
The programs must have unique names.
Reimplemented from Vamp::PluginBase.
|
virtualinherited |
Get the current program.
Reimplemented from Vamp::PluginBase.
|
virtualinherited |
Select a program.
(If the given program name is not one of the available programs, do nothing.)
Reimplemented from Vamp::PluginBase.
|
virtualinherited |
Get the minimum supported number of input channels.
Reimplemented from Vamp::Plugin.
|
virtualinherited |
Get the maximum supported number of input channels.
Reimplemented from Vamp::Plugin.
|
virtualinherited |
Get the outputs of this plugin.
An output's index in this list is used as its numeric index when looking it up in the FeatureSet returned from the process() call.
Implements Vamp::Plugin.
|
virtualinherited |
After all blocks have been processed, calculate and return any remaining features derived from the complete input.
Implements Vamp::Plugin.
|
inlineinherited |
Return a pointer to the plugin wrapper of type WrapperType surrounding this wrapper's plugin, if present.
This is useful in situations where a plugin is wrapped by multiple different wrappers (one inside another) and the host wants to call some wrapper-specific function on one of the layers without having to care about the order in which they are wrapped. For example, the plugin returned by PluginLoader::loadPlugin may have more than one wrapper; if the host wanted to query or fine-tune some property of one of them, it would be hard to do so without knowing the order of the wrappers. This function therefore gives direct access to the wrapper of a particular type.
Definition at line 119 of file PluginWrapper.h.
References Vamp::HostExt::PluginWrapper::getWrapper().
Referenced by Vamp::HostExt::PluginWrapper::getWrapper(), and runPlugin().
|
inherited |
Disown the wrapped plugin, so that we no longer delete it on our own destruction.
The identity of the wrapped plugin is unchanged, but this switches the expectation about ownership so that the caller is expected to handle the lifecycle of the wrapped plugin and to ensure that it outlasts this wrapper.
This is not the normal model, but could be useful in situations where a plugin is already managed using a smart pointer model before being adapted into this wrapper. Note that this can't be reversed - if you call this, you must subsequently take charge of the wrapped plugin yourself.
|
inlinevirtualinherited |
Used to distinguish between Vamp::Plugin and other potential sibling subclasses of PluginBase.
Do not reimplement this function in your subclass.
Implements Vamp::PluginBase.
Definition at line 438 of file vamp-sdk/Plugin.h.
|
inlineinherited |
Retrieve the input sample rate set on construction.
Definition at line 443 of file vamp-sdk/Plugin.h.
Member Data Documentation
|
protected |
Definition at line 227 of file PluginInputDomainAdapter.h.
|
protectedinherited |
Definition at line 144 of file PluginWrapper.h.
|
protectedinherited |
Definition at line 145 of file PluginWrapper.h.
|
protectedinherited |
Definition at line 449 of file vamp-sdk/Plugin.h.
Referenced by ZeroCrossing::getOutputDescriptors(), PercussionOnsetDetector::getOutputDescriptors(), AmplitudeFollower::initialise(), SpectralCentroid::process(), ZeroCrossing::process(), and PercussionOnsetDetector::process().
The documentation for this class was generated from the following file:
Generated by
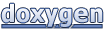