VampPluginSDK
2.10
|
#include <Plugin.h>
Public Types | |
enum | SampleType { OneSamplePerStep, FixedSampleRate, VariableSampleRate } |
Public Member Functions | |
OutputDescriptor () | |
Public Attributes | |
std::string | identifier |
The name of the output, in computer-usable form. More... | |
std::string | name |
The human-readable name of the output. More... | |
std::string | description |
A human-readable short text describing the output. More... | |
std::string | unit |
The unit of the output, in human-readable form. More... | |
bool | hasFixedBinCount |
True if the output has the same number of values per sample for every output sample. More... | |
size_t | binCount |
The number of values per result of the output. More... | |
std::vector< std::string > | binNames |
The (human-readable) names of each of the bins, if appropriate. More... | |
bool | hasKnownExtents |
True if the results in each output bin fall within a fixed numeric range (minimum and maximum values). More... | |
float | minValue |
Minimum value of the results in the output. More... | |
float | maxValue |
Maximum value of the results in the output. More... | |
bool | isQuantized |
True if the output values are quantized to a particular resolution. More... | |
float | quantizeStep |
Quantization resolution of the output values (e.g. More... | |
SampleType | sampleType |
Positioning in time of the output results. More... | |
float | sampleRate |
Sample rate of the output results, as samples per second. More... | |
bool | hasDuration |
True if the returned results for this output are known to have a duration field. More... | |
Detailed Description
Definition at line 206 of file vamp-sdk/Plugin.h.
Member Enumeration Documentation
Enumerator | |
---|---|
OneSamplePerStep |
Results from each process() align with that call's block start. |
FixedSampleRate |
Results are evenly spaced in time (sampleRate specified below) |
VariableSampleRate |
Results are unevenly spaced and have individual timestamps. |
Definition at line 287 of file vamp-sdk/Plugin.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 322 of file vamp-sdk/Plugin.h.
Member Data Documentation
std::string Vamp::Plugin::OutputDescriptor::identifier |
The name of the output, in computer-usable form.
Should be reasonably short and without whitespace or punctuation, using the characters [a-zA-Z0-9_-] only. Example: "zero_crossing_count"
Definition at line 214 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
std::string Vamp::Plugin::OutputDescriptor::name |
The human-readable name of the output.
Example: "Zero Crossing Counts"
Definition at line 220 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
std::string Vamp::Plugin::OutputDescriptor::description |
A human-readable short text describing the output.
May be empty if the name has said it all already. Example: "The number of zero crossing points per processing block"
Definition at line 227 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
std::string Vamp::Plugin::OutputDescriptor::unit |
The unit of the output, in human-readable form.
Definition at line 232 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
bool Vamp::Plugin::OutputDescriptor::hasFixedBinCount |
True if the output has the same number of values per sample for every output sample.
Outputs for which this is false are unlikely to be very useful in a general-purpose host.
Definition at line 239 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
size_t Vamp::Plugin::OutputDescriptor::binCount |
The number of values per result of the output.
Undefined if hasFixedBinCount is false. If this is zero, the output is point data (i.e. only the time of each output is of interest, the value list will be empty).
Definition at line 247 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
std::vector<std::string> Vamp::Plugin::OutputDescriptor::binNames |
The (human-readable) names of each of the bins, if appropriate.
This is always optional.
Definition at line 253 of file vamp-sdk/Plugin.h.
bool Vamp::Plugin::OutputDescriptor::hasKnownExtents |
True if the results in each output bin fall within a fixed numeric range (minimum and maximum values).
Undefined if binCount is zero.
Definition at line 260 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
float Vamp::Plugin::OutputDescriptor::minValue |
Minimum value of the results in the output.
Undefined if hasKnownExtents is false or binCount is zero.
Definition at line 266 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors().
float Vamp::Plugin::OutputDescriptor::maxValue |
Maximum value of the results in the output.
Undefined if hasKnownExtents is false or binCount is zero.
Definition at line 272 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors().
bool Vamp::Plugin::OutputDescriptor::isQuantized |
True if the output values are quantized to a particular resolution.
Undefined if binCount is zero.
Definition at line 278 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
float Vamp::Plugin::OutputDescriptor::quantizeStep |
Quantization resolution of the output values (e.g.
1.0 if they are all integers). Undefined if isQuantized is false or binCount is zero.
Definition at line 285 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
SampleType Vamp::Plugin::OutputDescriptor::sampleType |
Positioning in time of the output results.
Definition at line 302 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), AmplitudeFollower::getOutputDescriptors(), SpectralCentroid::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), PowerSpectrum::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
float Vamp::Plugin::OutputDescriptor::sampleRate |
Sample rate of the output results, as samples per second.
Undefined if sampleType is OneSamplePerStep.
If sampleType is VariableSampleRate and this value is non-zero, then it may be used to calculate a resolution for the output (i.e. the "duration" of each sample, in time, will be 1/sampleRate seconds). It's recommended to set this to zero if that behaviour is not desired.
Definition at line 314 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors(), ZeroCrossing::getOutputDescriptors(), and PercussionOnsetDetector::getOutputDescriptors().
bool Vamp::Plugin::OutputDescriptor::hasDuration |
True if the returned results for this output are known to have a duration field.
Definition at line 320 of file vamp-sdk/Plugin.h.
Referenced by FixedTempoEstimator::D::getOutputDescriptors().
The documentation for this struct was generated from the following file:
Generated by
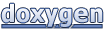