VampPluginSDK
2.10
|
PowerSpectrum.cpp
Go to the documentation of this file.
std::string getCopyright() const
Get the copyright statement or licensing summary for the plugin.
Definition: PowerSpectrum.cpp:86
bool hasFixedBinCount
True if the output has the same number of values per sample for every output sample.
Definition: vamp-sdk/Plugin.h:239
std::vector< float > values
Results for a single sample of this feature.
Definition: vamp-sdk/Plugin.h:382
OutputList getOutputDescriptors() const
Get the outputs of this plugin.
Definition: PowerSpectrum.cpp:108
std::string getName() const
Get a human-readable name or title of the plugin.
Definition: PowerSpectrum.cpp:62
std::string description
A human-readable short text describing the output.
Definition: vamp-sdk/Plugin.h:227
std::string identifier
The name of the output, in computer-usable form.
Definition: vamp-sdk/Plugin.h:214
FeatureSet process(const float *const *inputBuffers, Vamp::RealTime timestamp)
Process a single block of input data.
Definition: PowerSpectrum.cpp:136
Definition: vamp-sdk/Plugin.h:344
std::string getIdentifier() const
Get the computer-usable name of the plugin.
Definition: PowerSpectrum.cpp:56
std::string getDescription() const
Get a human-readable description for the plugin, typically a line of text that may optionally be disp...
Definition: PowerSpectrum.cpp:68
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: vamp-sdk/RealTime.h:66
bool initialise(size_t channels, size_t stepSize, size_t blockSize)
Initialise a plugin to prepare it for use with the given number of input channels, step size (window increment, in sample frames) and block size (window size, in sample frames).
Definition: PowerSpectrum.cpp:92
FeatureSet getRemainingFeatures()
After all blocks have been processed, calculate and return any remaining features derived from the co...
Definition: PowerSpectrum.cpp:166
Results from each process() align with that call's block start.
Definition: vamp-sdk/Plugin.h:290
virtual size_t getMaxChannelCount() const
Get the maximum supported number of input channels.
Definition: vamp-sdk/Plugin.h:204
std::string getMaker() const
Get the name of the author or vendor of the plugin in human-readable form.
Definition: PowerSpectrum.cpp:74
void reset()
Reset the plugin after use, to prepare it for another clean run.
Definition: PowerSpectrum.cpp:103
bool isQuantized
True if the output values are quantized to a particular resolution.
Definition: vamp-sdk/Plugin.h:278
virtual size_t getMinChannelCount() const
Get the minimum supported number of input channels.
Definition: vamp-sdk/Plugin.h:199
bool hasKnownExtents
True if the results in each output bin fall within a fixed numeric range (minimum and maximum values)...
Definition: vamp-sdk/Plugin.h:260
Generated by
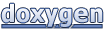