VampPluginSDK
2.10
|
ZeroCrossing.cpp
Go to the documentation of this file.
FeatureSet process(const float *const *inputBuffers, Vamp::RealTime timestamp)
Process a single block of input data.
Definition: ZeroCrossing.cpp:143
std::string getMaker() const
Get the name of the author or vendor of the plugin in human-readable form.
Definition: ZeroCrossing.cpp:77
float sampleRate
Sample rate of the output results, as samples per second.
Definition: vamp-sdk/Plugin.h:314
std::string getName() const
Get a human-readable name or title of the plugin.
Definition: ZeroCrossing.cpp:65
bool hasFixedBinCount
True if the output has the same number of values per sample for every output sample.
Definition: vamp-sdk/Plugin.h:239
std::string getIdentifier() const
Get the computer-usable name of the plugin.
Definition: ZeroCrossing.cpp:59
std::vector< float > values
Results for a single sample of this feature.
Definition: vamp-sdk/Plugin.h:382
bool initialise(size_t channels, size_t stepSize, size_t blockSize)
Initialise a plugin to prepare it for use with the given number of input channels, step size (window increment, in sample frames) and block size (window size, in sample frames).
Definition: ZeroCrossing.cpp:95
float quantizeStep
Quantization resolution of the output values (e.g.
Definition: vamp-sdk/Plugin.h:285
FeatureSet getRemainingFeatures()
After all blocks have been processed, calculate and return any remaining features derived from the co...
Definition: ZeroCrossing.cpp:192
std::string getDescription() const
Get a human-readable description for the plugin, typically a line of text that may optionally be disp...
Definition: ZeroCrossing.cpp:71
std::string description
A human-readable short text describing the output.
Definition: vamp-sdk/Plugin.h:227
void reset()
Reset the plugin after use, to prepare it for another clean run.
Definition: ZeroCrossing.cpp:106
std::string identifier
The name of the output, in computer-usable form.
Definition: vamp-sdk/Plugin.h:214
Definition: vamp-sdk/Plugin.h:344
OutputList getOutputDescriptors() const
Get the outputs of this plugin.
Definition: ZeroCrossing.cpp:112
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: vamp-sdk/RealTime.h:66
static RealTime frame2RealTime(long frame, unsigned int sampleRate)
Convert a sample frame at the given sample rate into a RealTime.
Results from each process() align with that call's block start.
Definition: vamp-sdk/Plugin.h:290
virtual size_t getMaxChannelCount() const
Get the maximum supported number of input channels.
Definition: vamp-sdk/Plugin.h:204
bool isQuantized
True if the output values are quantized to a particular resolution.
Definition: vamp-sdk/Plugin.h:278
virtual size_t getMinChannelCount() const
Get the minimum supported number of input channels.
Definition: vamp-sdk/Plugin.h:199
std::string getCopyright() const
Get the copyright statement or licensing summary for the plugin.
Definition: ZeroCrossing.cpp:89
Results are unevenly spaced and have individual timestamps.
Definition: vamp-sdk/Plugin.h:296
bool hasKnownExtents
True if the results in each output bin fall within a fixed numeric range (minimum and maximum values)...
Definition: vamp-sdk/Plugin.h:260
Generated by
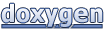