VampPluginSDK
2.10
|
A base class for plugins with optional configurable parameters, programs, etc. More...
#include <PluginBase.h>
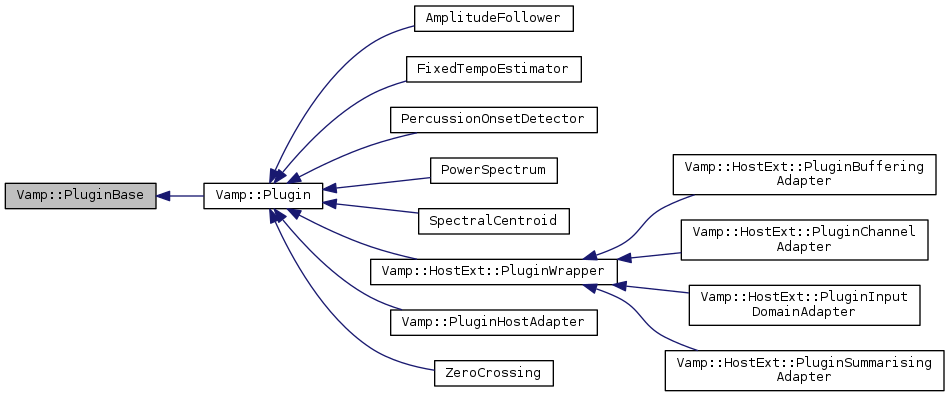
Classes | |
struct | ParameterDescriptor |
Public Types | |
typedef std::vector< ParameterDescriptor > | ParameterList |
typedef std::vector< std::string > | ProgramList |
Public Member Functions | |
virtual | ~PluginBase () |
virtual unsigned int | getVampApiVersion () const |
Get the Vamp API compatibility level of the plugin. More... | |
virtual std::string | getIdentifier () const =0 |
Get the computer-usable name of the plugin. More... | |
virtual std::string | getName () const =0 |
Get a human-readable name or title of the plugin. More... | |
virtual std::string | getDescription () const =0 |
Get a human-readable description for the plugin, typically a line of text that may optionally be displayed in addition to the plugin's "name". More... | |
virtual std::string | getMaker () const =0 |
Get the name of the author or vendor of the plugin in human-readable form. More... | |
virtual std::string | getCopyright () const =0 |
Get the copyright statement or licensing summary for the plugin. More... | |
virtual int | getPluginVersion () const =0 |
Get the version number of the plugin. More... | |
virtual ParameterList | getParameterDescriptors () const |
Get the controllable parameters of this plugin. More... | |
virtual float | getParameter (std::string) const |
Get the value of a named parameter. More... | |
virtual void | setParameter (std::string, float) |
Set a named parameter. More... | |
virtual ProgramList | getPrograms () const |
Get the program settings available in this plugin. More... | |
virtual std::string | getCurrentProgram () const |
Get the current program. More... | |
virtual void | selectProgram (std::string) |
Select a program. More... | |
virtual std::string | getType () const =0 |
Get the type of plugin. More... | |
Detailed Description
A base class for plugins with optional configurable parameters, programs, etc.
The Vamp::Plugin is derived from this, and individual Vamp plugins should derive from that.
This class does not provide the necessary interfaces to instantiate or run a plugin. It only specifies an interface for retrieving those controls that the host may wish to show to the user for editing. It could meaningfully be subclassed by real-time plugins or other sorts of plugin as well as Vamp plugins.
Definition at line 60 of file vamp-sdk/PluginBase.h.
Member Typedef Documentation
typedef std::vector<ParameterDescriptor> Vamp::PluginBase::ParameterList |
Definition at line 203 of file vamp-sdk/PluginBase.h.
typedef std::vector<std::string> Vamp::PluginBase::ProgramList |
Definition at line 225 of file vamp-sdk/PluginBase.h.
Constructor & Destructor Documentation
|
inlinevirtual |
Definition at line 63 of file vamp-sdk/PluginBase.h.
Member Function Documentation
|
inlinevirtual |
Get the Vamp API compatibility level of the plugin.
Reimplemented in Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 68 of file vamp-sdk/PluginBase.h.
Referenced by enumeratePlugins().
|
pure virtual |
Get the computer-usable name of the plugin.
This should be reasonably short and contain no whitespace or punctuation characters. It may only contain the characters [a-zA-Z0-9_-]. This is the authoritative way for a program to identify a plugin within a given library.
This text may be visible to the user, but it should not be the main text used to identify a plugin to the user (that will be the name, below).
Example: "zero_crossings"
Implemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, PowerSpectrum, AmplitudeFollower, SpectralCentroid, ZeroCrossing, FixedTempoEstimator, and PercussionOnsetDetector.
Referenced by enumeratePlugins(), and runPlugin().
|
pure virtual |
Get a human-readable name or title of the plugin.
This should be brief and self-contained, as it may be used to identify the plugin to the user in isolation (i.e. without also showing the plugin's "identifier").
Example: "Zero Crossings"
Implemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, PowerSpectrum, AmplitudeFollower, SpectralCentroid, ZeroCrossing, FixedTempoEstimator, and PercussionOnsetDetector.
Referenced by enumeratePlugins(), and printPluginCategoryList().
|
pure virtual |
Get a human-readable description for the plugin, typically a line of text that may optionally be displayed in addition to the plugin's "name".
May be empty if the name has said it all already.
Example: "Detect and count zero crossing points"
Implemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, PowerSpectrum, AmplitudeFollower, SpectralCentroid, ZeroCrossing, FixedTempoEstimator, and PercussionOnsetDetector.
Referenced by enumeratePlugins(), and printPluginCategoryList().
|
pure virtual |
Get the name of the author or vendor of the plugin in human-readable form.
This should be a short identifying text, as it may be used to label plugins from the same source in a menu or similar.
Implemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, PowerSpectrum, AmplitudeFollower, SpectralCentroid, ZeroCrossing, FixedTempoEstimator, and PercussionOnsetDetector.
Referenced by enumeratePlugins(), and printPluginCategoryList().
|
pure virtual |
Get the copyright statement or licensing summary for the plugin.
This can be an informative text, without the same presentation constraints as mentioned for getMaker above.
Implemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, PowerSpectrum, AmplitudeFollower, SpectralCentroid, ZeroCrossing, FixedTempoEstimator, and PercussionOnsetDetector.
Referenced by enumeratePlugins().
|
pure virtual |
Get the version number of the plugin.
Implemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, PowerSpectrum, AmplitudeFollower, SpectralCentroid, ZeroCrossing, FixedTempoEstimator, and PercussionOnsetDetector.
Referenced by enumeratePlugins().
|
inlinevirtual |
Get the controllable parameters of this plugin.
Reimplemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, AmplitudeFollower, FixedTempoEstimator, and PercussionOnsetDetector.
Definition at line 208 of file vamp-sdk/PluginBase.h.
Referenced by enumeratePlugins().
|
inlinevirtual |
Get the value of a named parameter.
The argument is the identifier field from that parameter's descriptor.
Reimplemented in Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, AmplitudeFollower, FixedTempoEstimator, and PercussionOnsetDetector.
Definition at line 216 of file vamp-sdk/PluginBase.h.
|
inlinevirtual |
Set a named parameter.
The first argument is the identifier field from that parameter's descriptor.
Reimplemented in Vamp::HostExt::PluginBufferingAdapter, Vamp::PluginHostAdapter, Vamp::HostExt::PluginWrapper, AmplitudeFollower, FixedTempoEstimator, and PercussionOnsetDetector.
Definition at line 222 of file vamp-sdk/PluginBase.h.
|
inlinevirtual |
Get the program settings available in this plugin.
A program is a named shorthand for a set of parameter values; changing the program may cause the plugin to alter the values of its published parameters (and/or non-public internal processing parameters). The host should re-read the plugin's parameter values after setting a new program.
The programs must have unique names.
Reimplemented in Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 237 of file vamp-sdk/PluginBase.h.
|
inlinevirtual |
Get the current program.
Reimplemented in Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 242 of file vamp-sdk/PluginBase.h.
|
inlinevirtual |
Select a program.
(If the given program name is not one of the available programs, do nothing.)
Reimplemented in Vamp::HostExt::PluginBufferingAdapter, Vamp::PluginHostAdapter, and Vamp::HostExt::PluginWrapper.
Definition at line 248 of file vamp-sdk/PluginBase.h.
References _VAMP_SDK_PLUGSPACE_END.
|
pure virtual |
Get the type of plugin.
This is to be implemented by the immediate subclass, not by actual plugins. Do not attempt to implement this in plugin code.
Implemented in Vamp::Plugin.
The documentation for this class was generated from the following file:
Generated by
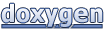