FFmpeg
|
#include "libavutil/imgutils.h"
#include "avcodec.h"
#include "internal.h"
#include "vp8.h"
#include "vp8data.h"
#include "rectangle.h"
#include "thread.h"
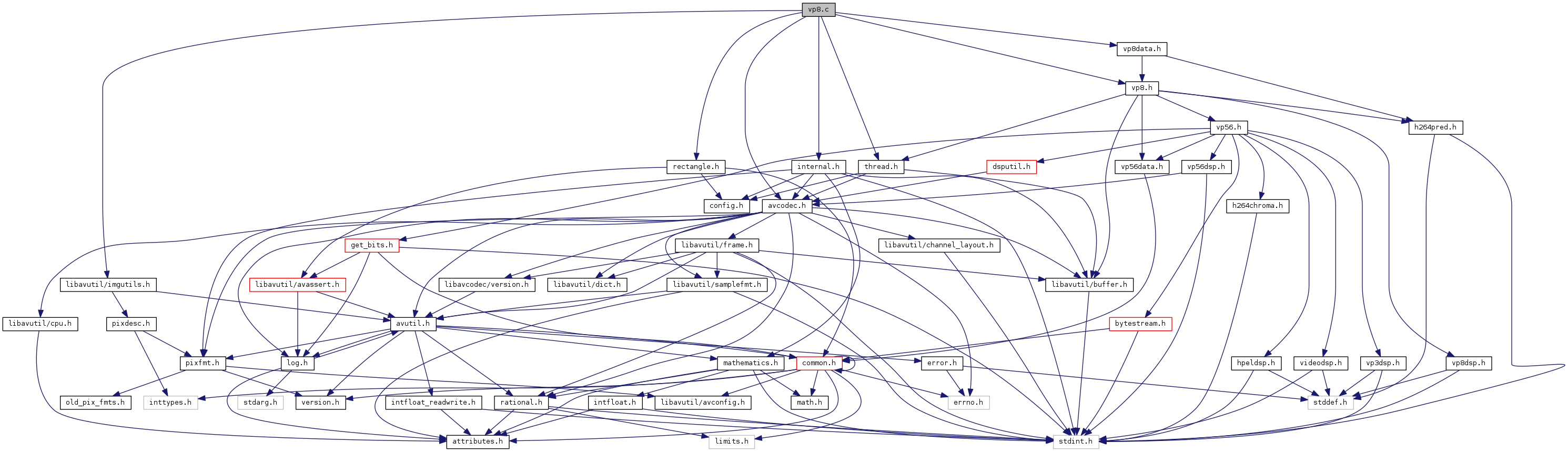
Go to the source code of this file.
Macros | |
#define | MV_EDGE_CHECK(n) |
#define | XCHG(a, b, xchg) |
#define | MARGIN (16 << 2) |
#define | check_thread_pos(td, otd, mb_x_check, mb_y_check) |
#define | update_pos(td, mb_y, mb_x) |
#define | REBASE(pic) pic ? pic - &s_src->frames[0] + &s->frames[0] : NULL |
Variables | |
static const uint8_t | subpel_idx [3][8] |
AVCodec | ff_vp8_decoder |
AVCodec | ff_webp_decoder |
Macro Definition Documentation
#define check_thread_pos | ( | td, | |
otd, | |||
mb_x_check, | |||
mb_y_check | |||
) |
Definition at line 1656 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter(), and vp8_filter_mb_row().
#define MARGIN (16 << 2) |
Definition at line 1592 of file vp8.c.
Referenced by vp8_decode_frame(), vp8_decode_mb_row_no_filter(), and vp8_decode_mv_mb_modes().
#define MV_EDGE_CHECK | ( | n | ) |
Referenced by decode_mvs().
Definition at line 2065 of file vp8.c.
Referenced by vp8_decode_update_thread_context().
#define update_pos | ( | td, | |
mb_y, | |||
mb_x | |||
) |
Definition at line 1657 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter(), vp8_decode_mb_row_sliced(), and vp8_filter_mb_row().
Referenced by xchg_mb_border().
Function Documentation
|
static |
Definition at line 2101 of file vp8.c.
Referenced by webp_decode_frame().
|
static |
Definition at line 903 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter(), and vp8_filter_mb_row().
|
static |
Definition at line 945 of file vp8.c.
Referenced by check_intra_pred8x8_mode(), and check_intra_pred8x8_mode_emuedge().
|
static |
Definition at line 1001 of file vp8.c.
Referenced by intra_predict().
|
static |
Definition at line 965 of file vp8.c.
Referenced by intra_predict().
|
static |
Definition at line 975 of file vp8.c.
Referenced by intra_predict().
|
static |
Definition at line 991 of file vp8.c.
Referenced by check_intra_pred4x4_mode_emuedge().
|
static |
Definition at line 955 of file vp8.c.
Referenced by check_intra_pred8x8_mode_emuedge().
|
static |
Definition at line 442 of file vp8.c.
Referenced by decode_mvs().
|
static |
- Parameters
-
c arithmetic bitstream reader context block destination for block coefficients probs probabilities to use when reading trees from the bitstream i initial coeff index, 0 unless a separate DC block is coded zero_nhood the initial prediction context for number of surrounding all-zero blocks (only left/top, so 0-2) qmul array holding the dc/ac dequant factor at position 0/1
- Returns
- 0 if no coeffs were decoded otherwise, the index of the last coeff decoded plus one
Definition at line 831 of file vp8.c.
Referenced by decode_mb_coeffs().
|
static |
- Parameters
-
r arithmetic bitstream reader context block destination for block coefficients probs probabilities to use when reading trees from the bitstream i initial coeff index, 0 unless a separate DC block is coded qmul array holding the dc/ac dequant factor at position 0/1
- Returns
- 0 if no coeffs were decoded otherwise, the index of the last coeff decoded plus one
Definition at line 763 of file vp8.c.
Referenced by decode_block_coeffs().
|
static |
Definition at line 304 of file vp8.c.
Referenced by vp8_decode_frame().
|
static |
Definition at line 664 of file vp8.c.
Referenced by decode_mb_mode().
|
static |
Definition at line 842 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter().
|
static |
Definition at line 698 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter(), and vp8_decode_mv_mb_modes().
|
static |
Definition at line 565 of file vp8.c.
Referenced by decode_mb_mode().
|
static |
Split motion vector prediction, 16.4.
- Returns
- the number of motion vectors parsed (2, 4 or 16)
Definition at line 494 of file vp8.c.
Referenced by decode_mvs().
|
static |
Definition at line 1461 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter().
|
static |
Definition at line 1491 of file vp8.c.
Referenced by vp8_filter_mb_row().
|
static |
Definition at line 1561 of file vp8.c.
Referenced by vp8_filter_mb_row().
|
static |
Definition at line 38 of file vp8.c.
Referenced by vp8_decode_flush_impl(), and vp8_decode_update_thread_context().
|
static |
Definition at line 231 of file vp8.c.
Referenced by decode_frame_header().
|
static |
Definition at line 480 of file vp8.c.
Referenced by decode_splitmvs().
|
static |
Definition at line 1408 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter().
|
static |
Apply motion vectors to prediction buffer, chapter 18.
Definition at line 1328 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter().
|
static |
Definition at line 1035 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter().
|
static |
Definition at line 160 of file vp8.c.
Referenced by decode_frame_header().
|
static |
Definition at line 1306 of file vp8.c.
Referenced by vp8_decode_mb_row_no_filter().
|
static |
Motion vector coding, 17.1.
Definition at line 451 of file vp8.c.
Referenced by decode_mvs(), and decode_splitmvs().
|
static |
Determine which buffers golden and altref should be updated with after this frame.
The spec isn't clear here, so I'm going by my understanding of what libvpx does
Intra frames update all 3 references Inter frames update VP56_FRAME_PREVIOUS if the update_last flag is set If the update (golden|altref) flag is set, it's updated with the current frame if update_last is set, and VP56_FRAME_PREVIOUS otherwise. If the flag is not set, the number read means: 0: no update 1: VP56_FRAME_PREVIOUS 2: update golden with altref, or update altref with golden
Definition at line 277 of file vp8.c.
Referenced by update_refs().
|
static |
Definition at line 205 of file vp8.c.
Referenced by decode_frame_header().
|
static |
Definition at line 114 of file vp8.c.
Referenced by decode_frame_header().
|
static |
Definition at line 181 of file vp8.c.
Referenced by decode_frame_header().
|
static |
Definition at line 293 of file vp8.c.
Referenced by decode_frame_header().
|
static |
Definition at line 59 of file vp8.c.
Referenced by vp8_decode_frame().
|
static |
|
static |
Definition at line 96 of file vp8.c.
Referenced by update_dimensions(), vp8_decode_flush(), and vp8_decode_free().
|
static |
Definition at line 1852 of file vp8.c.
Referenced by webp_decode_frame().
|
static |
Definition at line 2006 of file vp8.c.
Referenced by vp8_decode_init(), and vp8_decode_init_thread_copy().
|
static |
|
static |
|
static |
Definition at line 1660 of file vp8.c.
Referenced by vp8_decode_mb_row_sliced().
|
static |
Definition at line 1825 of file vp8.c.
Referenced by vp8_decode_frame().
|
static |
Definition at line 1593 of file vp8.c.
Referenced by vp8_decode_frame().
|
static |
|
static |
Definition at line 1771 of file vp8.c.
Referenced by vp8_decode_mb_row_sliced().
|
static |
Definition at line 2018 of file vp8.c.
Referenced by vp8_decode_init(), and vp8_decode_init_thread_copy().
|
static |
chroma MC function
- Parameters
-
s VP8 decoding context dst1 target buffer for block data at block position (U plane) dst2 target buffer for block data at block position (V plane) ref reference picture buffer at origin (0, 0) mv motion vector (relative to block position) to get pixel data from x_off horizontal position of block from origin (0, 0) y_off vertical position of block from origin (0, 0) block_w width of block (16, 8 or 4) block_h height of block (always same as block_w) width width of src/dst plane data height height of src/dst plane data linesize size of a single line of plane data, including padding mc_func motion compensation function pointers (bilinear or sixtap MC)
Definition at line 1231 of file vp8.c.
Referenced by inter_predict(), and vp8_mc_part().
|
static |
luma MC function
- Parameters
-
s VP8 decoding context dst target buffer for block data at block position ref reference picture buffer at origin (0, 0) mv motion vector (relative to block position) to get pixel data from x_off horizontal position of block from origin (0, 0) y_off vertical position of block from origin (0, 0) block_w width of block (16, 8 or 4) block_h height of block (always same as block_w) width width of src/dst plane data height height of src/dst plane data linesize size of a single line of plane data, including padding mc_func motion compensation function pointers (bilinear or sixtap MC)
Definition at line 1180 of file vp8.c.
Referenced by inter_predict(), and vp8_mc_part().
|
static |
Definition at line 1274 of file vp8.c.
Referenced by inter_predict().
|
static |
Definition at line 78 of file vp8.c.
Referenced by vp8_decode_update_thread_context().
|
static |
Definition at line 72 of file vp8.c.
Referenced by vp8_decode_flush_impl(), vp8_decode_frame(), and vp8_ref_frame().
|
static |
|
static |
Definition at line 914 of file vp8.c.
Referenced by intra_predict().
Variable Documentation
AVCodec ff_vp8_decoder |
AVCodec ff_webp_decoder |
|
static |
Definition at line 1156 of file vp8.c.
Referenced by vp8_mc_chroma(), and vp8_mc_luma().
Generated on Wed Apr 16 2025 06:53:30 for FFmpeg by
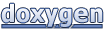