FFmpeg
|
AAC encoder. More...
#include "libavutil/float_dsp.h"
#include "libavutil/opt.h"
#include "avcodec.h"
#include "put_bits.h"
#include "internal.h"
#include "mpeg4audio.h"
#include "kbdwin.h"
#include "sinewin.h"
#include "aac.h"
#include "aactab.h"
#include "aacenc.h"
#include "psymodel.h"
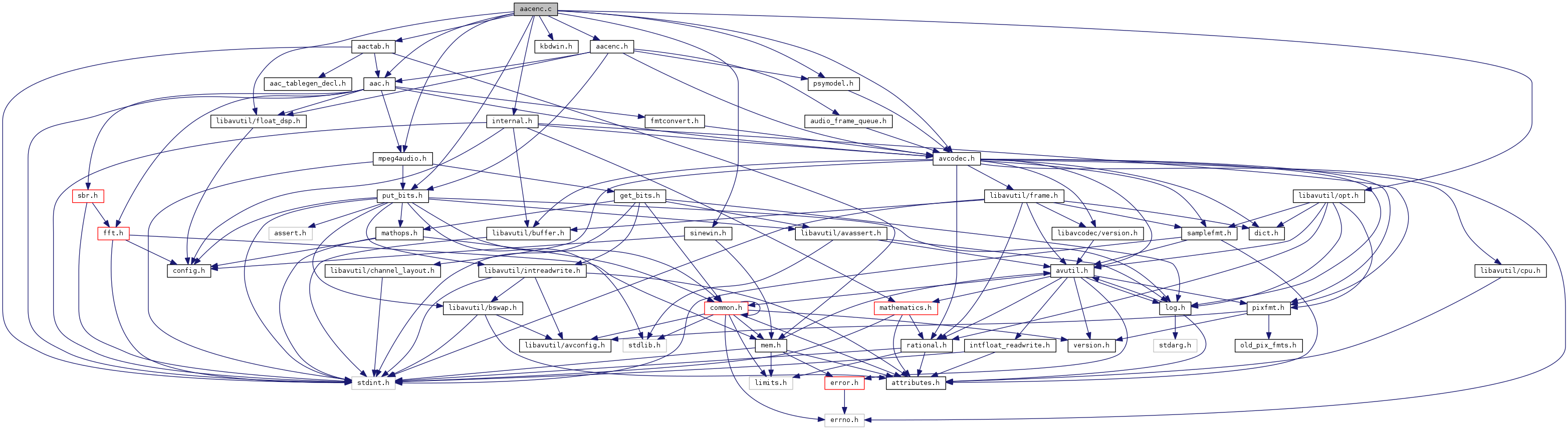
Go to the source code of this file.
Macros | |
#define | AAC_MAX_CHANNELS 6 |
#define | ERROR_IF(cond, ...) |
#define | WINDOW_FUNC(type) |
#define | AACENC_FLAGS AV_OPT_FLAG_ENCODING_PARAM | AV_OPT_FLAG_AUDIO_PARAM |
Detailed Description
AAC encoder.
Definition in file aacenc.c.
Macro Definition Documentation
#define AAC_MAX_CHANNELS 6 |
Definition at line 48 of file aacenc.c.
Referenced by aac_encode_frame(), and aac_encode_init().
#define AACENC_FLAGS AV_OPT_FLAG_ENCODING_PARAM | AV_OPT_FLAG_AUDIO_PARAM |
#define ERROR_IF | ( | cond, | |
... | |||
) |
Definition at line 50 of file aacenc.c.
Referenced by aac_encode_init().
#define WINDOW_FUNC | ( | type | ) |
Function Documentation
|
static |
Definition at line 670 of file aacenc.c.
Referenced by aac_encode_init().
|
static |
|
static |
|
static |
Produce integer coefficients from scalefactors provided by the model.
Definition at line 304 of file aacenc.c.
Referenced by aac_encode_frame().
|
static |
Definition at line 705 of file aacenc.c.
Referenced by aac_encode_init().
|
static |
Definition at line 249 of file aacenc.c.
Referenced by aac_encode_frame().
|
static |
Definition at line 482 of file aacenc.c.
Referenced by aac_encode_frame().
|
static |
Definition at line 685 of file aacenc.c.
Referenced by aac_encode_init().
|
static |
Encode scalefactor band coding type.
Definition at line 366 of file aacenc.c.
Referenced by encode_individual_channel().
|
static |
Encode one channel of audio data.
Definition at line 442 of file aacenc.c.
Referenced by aac_encode_frame().
|
static |
Encode MS data.
- See also
- 4.6.8.1 "Joint Coding - M/S Stereo"
Definition at line 290 of file aacenc.c.
Referenced by aac_encode_frame().
|
static |
Encode pulse data.
Definition at line 398 of file aacenc.c.
Referenced by encode_individual_channel().
|
static |
Encode scalefactors.
Definition at line 377 of file aacenc.c.
Referenced by encode_individual_channel().
|
static |
Encode spectral coefficients processed by psychoacoustic model.
Definition at line 417 of file aacenc.c.
Referenced by encode_individual_channel().
|
static |
Make AAC audio config object.
- See also
- 1.6.2.1 "Syntax - AudioSpecificConfig"
Definition at line 163 of file aacenc.c.
Referenced by aac_encode_init().
|
static |
Write some auxiliary information about the created AAC file.
Definition at line 461 of file aacenc.c.
Referenced by aac_encode_frame().
|
static |
Encode ics_info element.
- See also
- Table 4.6 (syntax of ics_info)
Definition at line 269 of file aacenc.c.
Referenced by aac_encode_frame(), and encode_individual_channel().
Variable Documentation
|
static |
default channel configurations
Definition at line 138 of file aacenc.c.
Referenced by aac_encode_init().
|
static |
Table to remap channels from libavcodec's default order to AAC order.
Definition at line 150 of file aacenc.c.
Referenced by copy_input_samples().
|
static |
|
static |
|
static |
Definition at line 240 of file aacenc.c.
Referenced by apply_window_and_mdct(), and ff_mpa_synth_filter().
AVCodec ff_aac_encoder |
float ff_aac_pow34sf_tab[428] |
Definition at line 56 of file aacenc.c.
Referenced by aac_encode_init(), and quantize_and_encode_band_cost_template().
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
Generated on Wed May 8 2024 06:52:15 for FFmpeg by
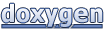