svgui
1.9
|
WaveformLayer.h
Go to the documentation of this file.
50 void paintVerticalScale(LayerGeometryProvider *v, bool detailed, QPainter &paint, QRect rect) const override;
PropertyType getPropertyType(const PropertyName &) const override
Definition: WaveformLayer.cpp:147
const ZoomConstraint * getZoomConstraint() const override
Return a zoom constraint object defining the supported zoom levels for this layer.
Definition: WaveformLayer.cpp:67
bool getDisplayExtents(double &min, double &max) const override
Return the minimum and maximum values within the visible area for the y axis of this layer...
Definition: WaveformLayer.cpp:399
bool isLayerScrollable(const LayerGeometryProvider *) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: WaveformLayer.cpp:490
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: WaveformLayer.cpp:575
double getMiddleLineHeight() const
Definition: WaveformLayer.h:147
Definition: WaveformLayer.h:31
Definition: SingleColourLayer.h:24
void setChannel(int)
Specify the channel to use from the source model.
Definition: WaveformLayer.cpp:308
bool getYScaleValue(const LayerGeometryProvider *v, int y, double &value, QString &unit) const override
Return the value and unit at the given y coordinate in the given view.
Definition: WaveformLayer.cpp:1432
int getChannelArrangement(int &min, int &max, bool &merging, bool &mixing) const
Return value is number of channels displayed.
Definition: WaveformLayer.cpp:446
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: WaveformLayer.cpp:166
void setMiddleLineHeight(double)
Specify the height of the middle of the waveform track or tracks within the layer, from 0.0 to 1.0.
Definition: WaveformLayer.cpp:328
Definition: Layer.h:352
Definition: WaveformLayer.h:117
int getYForValue(const LayerGeometryProvider *v, double value, int channel) const
Definition: WaveformLayer.cpp:1343
ColourSignificance getLayerColourSignificance() const override
Implements Layer::getLayerColourSignificance()
Definition: WaveformLayer.h:45
void setAutoNormalize(bool)
Toggle automatic normalization of the currently visible waveform.
Definition: WaveformLayer.cpp:281
int getVerticalZoomSteps(int &defaultStep) const override
Get the number of vertical zoom steps available for this layer.
Definition: WaveformLayer.cpp:1703
QString getPropertyLabel(const PropertyName &) const override
Definition: WaveformLayer.cpp:130
Definition: WaveformLayer.h:90
std::vector< RangeSummarisableTimeValueModel::RangeBlock > RangeVec
Definition: WaveformLayer.h:201
PropertyList getProperties() const override
Definition: WaveformLayer.cpp:116
QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: WaveformLayer.cpp:1255
void getOversampledRanges(int minChannel, int maxChannel, bool mixingOrMerging, sv_frame_t f0, sv_frame_t f1, int oversampleBy, RangeVec &ranges) const
Definition: WaveformLayer.cpp:774
bool getYScaleDifference(const LayerGeometryProvider *v, int y0, int y1, double &diff, QString &unit) const override
Return the difference between the values at the given y coordinates in the given view, and the unit of the difference.
Definition: WaveformLayer.cpp:1458
bool getAggressiveCacheing() const
Definition: WaveformLayer.h:168
QString getPropertyIconName(const PropertyName &) const override
Definition: WaveformLayer.cpp:140
void flagBaseColourChanged() override
Definition: WaveformLayer.h:234
void paintVerticalScale(LayerGeometryProvider *v, bool detailed, QPainter &paint, QRect rect) const override
Definition: WaveformLayer.cpp:1512
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
void setVerticalZoomStep(int) override
Set the vertical zoom step.
Definition: WaveformLayer.cpp:1719
void setProperty(const PropertyName &, int value) override
Definition: WaveformLayer.cpp:248
void setGain(float gain)
Set the gain multiplier for sample values in this view.
Definition: WaveformLayer.cpp:271
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: WaveformLayer.cpp:1634
bool getSourceFramesForX(LayerGeometryProvider *v, int x, int modelZoomLevel, sv_frame_t &f0, sv_frame_t &f1) const
Definition: WaveformLayer.cpp:499
int getCompletion(LayerGeometryProvider *) const override
Return the proportion of background work complete in drawing this view, as a percentage – in most ca...
Definition: WaveformLayer.cpp:346
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: WaveformLayer.cpp:216
void paintChannelScaleGuides(LayerGeometryProvider *, QPainter *paint, QRect rect, int channel) const
Definition: WaveformLayer.cpp:1195
ModelId getModel() const override
Return the ID of the model represented in this layer.
Definition: WaveformLayer.h:40
RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: WaveformLayer.cpp:239
int getCurrentVerticalZoomStep() const override
Get the current vertical zoom step.
Definition: WaveformLayer.cpp:1710
float getNormalizeGain(LayerGeometryProvider *v, int channel) const
Definition: WaveformLayer.cpp:530
QString getPropertyGroupName(const PropertyName &) const override
Definition: WaveformLayer.cpp:157
bool canExistWithoutModel() const override
Return true if this layer type can function without a model being set.
Definition: WaveformLayer.h:194
int getVerticalScaleWidth(LayerGeometryProvider *v, bool detailed, QPainter &) const override
Definition: WaveformLayer.cpp:1496
void paintChannel(LayerGeometryProvider *, QPainter *paint, QRect rect, int channel, const RangeVec &ranges, int blockSize, sv_frame_t frame0, sv_frame_t frame1) const
Definition: WaveformLayer.cpp:849
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
Definition: WaveformLayer.h:117
void getSummaryRanges(int minChannel, int maxChannel, bool mixingOrMerging, sv_frame_t f0, sv_frame_t f1, int blockSize, RangeVec &ranges) const
Definition: WaveformLayer.cpp:742
Definition: WaveformLayer.h:90
void setShowMeans(bool)
Set whether to display mean values as a lighter-coloured area beneath the peaks.
Definition: WaveformLayer.cpp:290
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: WaveformLayer.cpp:1667
void setAggressiveCacheing(bool)
Enable or disable aggressive pixmap cacheing.
Definition: WaveformLayer.cpp:337
bool getValueExtents(double &min, double &max, bool &log, QString &unit) const override
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: WaveformLayer.cpp:356
Definition: WaveformLayer.h:117
void setChannelMode(ChannelMode)
Specify whether multi-channel audio data should be displayed with a separate axis per channel (Separa...
Definition: WaveformLayer.cpp:299
double getValueForY(const LayerGeometryProvider *v, int y, int &channel) const
Definition: WaveformLayer.cpp:1386
Generated by
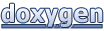