svgui
1.9
|
TimeRulerLayer.h
Go to the documentation of this file.
57 int getVerticalScaleWidth(LayerGeometryProvider *, bool, QPainter &) const override { return 0; }
Definition: TimeRulerLayer.h:40
int getXForUSec(LayerGeometryProvider *, double usec) const
Definition: TimeRulerLayer.cpp:217
Definition: Layer.h:350
int getDefaultColourHint(bool dark, bool &impose) override
Definition: TimeRulerLayer.cpp:425
Definition: SingleColourLayer.h:24
ModelId getModel() const override
Return the ID of the model represented in this layer.
Definition: TimeRulerLayer.h:38
Definition: TimeRulerLayer.h:40
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: TimeRulerLayer.cpp:252
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: TimeRulerLayer.cpp:441
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: TimeRulerLayer.cpp:448
Definition: TimeRulerLayer.h:40
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
Definition: TimeRulerLayer.h:28
ColourSignificance getLayerColourSignificance() const override
Implements Layer::getLayerColourSignificance()
Definition: TimeRulerLayer.h:47
bool snapToFeatureFrame(LayerGeometryProvider *, sv_frame_t &, int &, SnapType, int) const override
Adjust the given frame to snap to the nearest feature, if possible.
Definition: TimeRulerLayer.cpp:53
QString getLayerPresentationName() const override
Definition: TimeRulerLayer.cpp:432
int getVerticalScaleWidth(LayerGeometryProvider *, bool, QPainter &) const override
Definition: TimeRulerLayer.h:57
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
bool getValueExtents(double &, double &, bool &, QString &) const override
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: TimeRulerLayer.h:51
bool canExistWithoutModel() const override
Return true if this layer type can function without a model being set.
Definition: TimeRulerLayer.h:64
int64_t getMajorTickUSec(LayerGeometryProvider *, bool &quarterTicks) const
Definition: TimeRulerLayer.cpp:136
Generated by
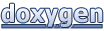