FFmpeg
|
WavPack lossless audio decoder. More...
#include "libavutil/channel_layout.h"
#include "avcodec.h"
#include "get_bits.h"
#include "internal.h"
#include "unary.h"
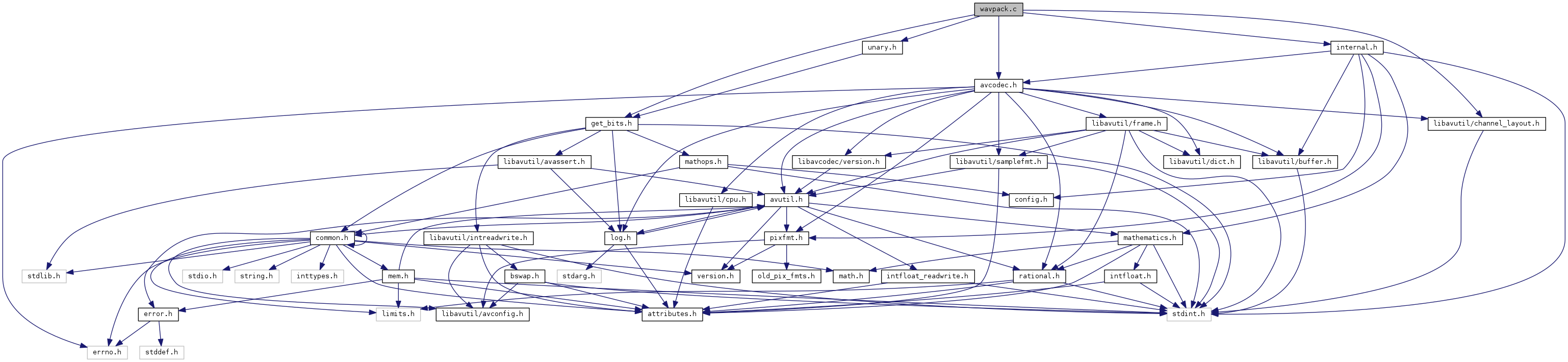
Go to the source code of this file.
Data Structures | |
struct | SavedContext |
struct | Decorr |
struct | WvChannel |
struct | WavpackFrameContext |
struct | WavpackContext |
Macros | |
#define | BITSTREAM_READER_LE |
#define | WV_MONO 0x00000004 |
#define | WV_JOINT_STEREO 0x00000010 |
#define | WV_FALSE_STEREO 0x40000000 |
#define | WV_HYBRID_MODE 0x00000008 |
#define | WV_HYBRID_SHAPE 0x00000008 |
#define | WV_HYBRID_BITRATE 0x00000200 |
#define | WV_HYBRID_BALANCE 0x00000400 |
#define | WV_FLT_SHIFT_ONES 0x01 |
#define | WV_FLT_SHIFT_SAME 0x02 |
#define | WV_FLT_SHIFT_SENT 0x04 |
#define | WV_FLT_ZERO_SENT 0x08 |
#define | WV_FLT_ZERO_SIGN 0x10 |
#define | WV_MAX_SAMPLES 131072 |
#define | MAX_TERMS 16 |
#define | WV_MAX_FRAME_DECODERS 14 |
#define | LEVEL_DECAY(a) ((a + 0x80) >> 8) |
#define | GET_MED(n) ((c->median[n] >> 4) + 1) |
#define | DEC_MED(n) c->median[n] -= ((c->median[n] + (128 >> n) - 2) / (128 >> n)) * 2 |
#define | INC_MED(n) c->median[n] += ((c->median[n] + (128 >> n) ) / (128 >> n)) * 5 |
#define | UPDATE_WEIGHT_CLIP(weight, delta, samples, in) |
Typedefs | |
typedef struct SavedContext | SavedContext |
typedef struct Decorr | Decorr |
typedef struct WvChannel | WvChannel |
typedef struct WavpackFrameContext | WavpackFrameContext |
typedef struct WavpackContext | WavpackContext |
Enumerations | |
enum | WP_ID_Flags { WP_IDF_MASK = 0x1F, WP_IDF_IGNORE = 0x20, WP_IDF_ODD = 0x40, WP_IDF_LONG = 0x80 } |
enum | WP_ID { WP_ID_DUMMY = 0, WP_ID_ENCINFO, WP_ID_DECTERMS, WP_ID_DECWEIGHTS, WP_ID_DECSAMPLES, WP_ID_ENTROPY, WP_ID_HYBRID, WP_ID_SHAPING, WP_ID_FLOATINFO, WP_ID_INT32INFO, WP_ID_DATA, WP_ID_CORR, WP_ID_EXTRABITS, WP_ID_CHANINFO } |
Variables | |
static const uint8_t | wp_exp2_table [256] |
static const uint8_t | wp_log2_table [] |
AVCodec | ff_wavpack_decoder |
Detailed Description
WavPack lossless audio decoder.
Definition in file wavpack.c.
Macro Definition Documentation
Definition at line 217 of file wavpack.c.
Referenced by wv_get_value().
Definition at line 216 of file wavpack.c.
Referenced by wv_get_value().
Definition at line 218 of file wavpack.c.
Referenced by wv_get_value().
Definition at line 213 of file wavpack.c.
Referenced by update_error_limit(), and wv_get_value().
#define MAX_TERMS 16 |
Definition at line 83 of file wavpack.c.
Referenced by wavpack_decode_block().
Definition at line 221 of file wavpack.c.
Referenced by wv_unpack_stereo().
#define WV_FALSE_STEREO 0x40000000 |
Definition at line 37 of file wavpack.c.
Referenced by wavpack_decode_block().
#define WV_FLT_SHIFT_ONES 0x01 |
Definition at line 44 of file wavpack.c.
Referenced by wv_get_value_float().
#define WV_FLT_SHIFT_SAME 0x02 |
Definition at line 45 of file wavpack.c.
Referenced by wv_get_value_float().
#define WV_FLT_SHIFT_SENT 0x04 |
Definition at line 46 of file wavpack.c.
Referenced by wv_get_value_float().
#define WV_FLT_ZERO_SENT 0x08 |
Definition at line 47 of file wavpack.c.
Referenced by wv_get_value_float().
#define WV_FLT_ZERO_SIGN 0x10 |
Definition at line 48 of file wavpack.c.
Referenced by wv_get_value_float().
#define WV_HYBRID_BITRATE 0x00000200 |
Definition at line 41 of file wavpack.c.
Referenced by wavpack_decode_block().
#define WV_HYBRID_MODE 0x00000008 |
Definition at line 39 of file wavpack.c.
Referenced by wavpack_decode_block().
#define WV_JOINT_STEREO 0x00000010 |
Definition at line 36 of file wavpack.c.
Referenced by wavpack_decode_block().
#define WV_MAX_FRAME_DECODERS 14 |
Definition at line 128 of file wavpack.c.
Referenced by wv_alloc_frame_context().
#define WV_MAX_SAMPLES 131072 |
Definition at line 50 of file wavpack.c.
Referenced by wavpack_decode_frame().
#define WV_MONO 0x00000004 |
Definition at line 35 of file wavpack.c.
Referenced by wavpack_decode_block().
Typedef Documentation
typedef struct SavedContext SavedContext |
typedef struct WavpackContext WavpackContext |
typedef struct WavpackFrameContext WavpackFrameContext |
Enumeration Type Documentation
enum WP_ID |
enum WP_ID_Flags |
Function Documentation
|
static |
Definition at line 235 of file wavpack.c.
Referenced by wv_get_value().
|
static |
Definition at line 249 of file wavpack.c.
Referenced by wv_get_value().
|
static |
Definition at line 762 of file wavpack.c.
Referenced by wavpack_decode_frame().
|
static |
|
static |
Definition at line 1171 of file wavpack.c.
Referenced by wavpack_decode_frame().
|
static |
|
static |
|
static |
Definition at line 182 of file wavpack.c.
Referenced by update_error_limit(), and wavpack_decode_block().
|
static |
Definition at line 197 of file wavpack.c.
Referenced by wv_get_value().
|
static |
Definition at line 706 of file wavpack.c.
Referenced by wavpack_decode_block().
|
inlinestatic |
Definition at line 509 of file wavpack.c.
Referenced by wv_unpack_mono(), and wv_unpack_stereo().
|
static |
Definition at line 283 of file wavpack.c.
Referenced by wv_unpack_mono(), and wv_unpack_stereo().
|
static |
Definition at line 430 of file wavpack.c.
Referenced by wv_unpack_mono(), and wv_unpack_stereo().
|
inlinestatic |
Definition at line 407 of file wavpack.c.
Referenced by wv_unpack_mono(), and wv_unpack_stereo().
|
static |
Definition at line 503 of file wavpack.c.
Referenced by wavpack_decode_flush(), wv_alloc_frame_context(), wv_unpack_mono(), and wv_unpack_stereo().
|
inlinestatic |
Definition at line 641 of file wavpack.c.
Referenced by wavpack_decode_block().
|
inlinestatic |
Definition at line 524 of file wavpack.c.
Referenced by wavpack_decode_block().
Variable Documentation
AVCodec ff_wavpack_decoder |
|
static |
Definition at line 144 of file wavpack.c.
Referenced by wp_exp2().
|
static |
Definition at line 163 of file wavpack.c.
Referenced by wp_log2().
Generated on Wed Apr 16 2025 06:53:30 for FFmpeg by
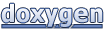