FFmpeg
|
Fieldmatching filter, ported from VFM filter (VapouSsynth) by Clément. More...
#include <inttypes.h>
#include "libavutil/avassert.h"
#include "libavutil/imgutils.h"
#include "libavutil/opt.h"
#include "libavutil/timestamp.h"
#include "avfilter.h"
#include "internal.h"
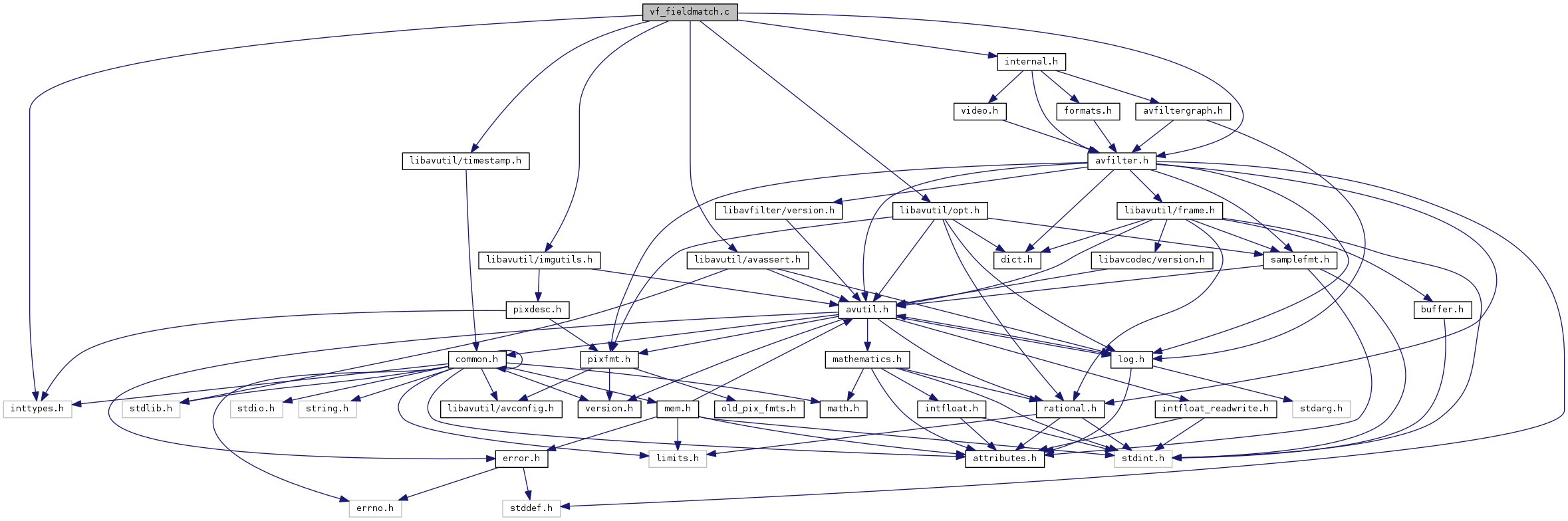
Go to the source code of this file.
Data Structures | |
struct | FieldMatchContext |
Macros | |
#define | INPUT_MAIN 0 |
#define | INPUT_CLEANSRC 1 |
#define | OFFSET(x) offsetof(FieldMatchContext, x) |
#define | FLAGS AV_OPT_FLAG_VIDEO_PARAM|AV_OPT_FLAG_FILTERING_PARAM |
#define | FILTER(xm2, xm1, xp1, xp2) |
#define | HAS_FF_AROUND(p, lz) |
#define | C_ARRAY_ADD(v) |
#define | VERTICAL_HALF(y_start, y_end) |
#define | LOAD_COMB(mid) |
#define | SLIDING_FRAME_WINDOW(prv, src, nxt) |
Enumerations | |
enum | fieldmatch_parity { FM_PARITY_AUTO = -1, FM_PARITY_BOTTOM = 0, FM_PARITY_TOP = 1 } |
enum | matching_mode { MODE_PC, MODE_PC_N, MODE_PC_U, MODE_PC_N_UB, MODE_PCN, MODE_PCN_UB, NB_MODE } |
enum | comb_matching_mode { COMBMATCH_NONE, COMBMATCH_SC, COMBMATCH_FULL, NB_COMBMATCH } |
enum | comb_dbg { COMBDBG_NONE, COMBDBG_PCN, COMBDBG_PCNUB, NB_COMBDBG } |
enum | { mP, mC, mN, mB, mU } |
Functions | |
AVFILTER_DEFINE_CLASS (fieldmatch) | |
static int | get_width (const FieldMatchContext *fm, const AVFrame *f, int plane) |
static int | get_height (const FieldMatchContext *fm, const AVFrame *f, int plane) |
static int64_t | luma_abs_diff (const AVFrame *f1, const AVFrame *f2) |
static void | fill_buf (uint8_t *data, int w, int h, int linesize, uint8_t v) |
static int | calc_combed_score (const FieldMatchContext *fm, const AVFrame *src) |
static void | build_abs_diff_mask (const uint8_t *prvp, int prv_linesize, const uint8_t *nxtp, int nxt_linesize, uint8_t *tbuffer, int tbuf_linesize, int width, int height) |
static void | build_diff_map (FieldMatchContext *fm, const uint8_t *prvp, int prv_linesize, const uint8_t *nxtp, int nxt_linesize, uint8_t *dstp, int dst_linesize, int height, int width, int plane) |
Build a map over which pixels differ a lot/a little. More... | |
static int | get_field_base (int match, int field) |
static AVFrame * | select_frame (FieldMatchContext *fm, int match) |
static int | compare_fields (FieldMatchContext *fm, int match1, int match2, int field) |
static void | copy_fields (const FieldMatchContext *fm, AVFrame *dst, const AVFrame *src, int field) |
static AVFrame * | create_weave_frame (AVFilterContext *ctx, int match, int field, const AVFrame *prv, AVFrame *src, const AVFrame *nxt) |
static int | checkmm (AVFilterContext *ctx, int *combs, int m1, int m2, AVFrame **gen_frames, int field) |
static int | filter_frame (AVFilterLink *inlink, AVFrame *in) |
static int | request_inlink (AVFilterContext *ctx, int lid) |
static int | request_frame (AVFilterLink *outlink) |
static int | query_formats (AVFilterContext *ctx) |
static int | config_input (AVFilterLink *inlink) |
static av_cold int | fieldmatch_init (AVFilterContext *ctx) |
static av_cold void | fieldmatch_uninit (AVFilterContext *ctx) |
static int | config_output (AVFilterLink *outlink) |
Variables | |
static const AVOption | fieldmatch_options [] |
static const int | fxo0m [] = { mP, mC, mN, mB, mU } |
static const int | fxo1m [] = { mN, mC, mP, mU, mB } |
static const AVFilterPad | fieldmatch_outputs [] |
AVFilter | avfilter_vf_fieldmatch |
Detailed Description
Fieldmatching filter, ported from VFM filter (VapouSsynth) by Clément.
Fredrik Mellbin is the author of the VIVTC/VFM filter, which is itself a light clone of the TIVTC/TFM (AviSynth) filter written by Kevin Stone (tritical), the original author.
Definition in file vf_fieldmatch.c.
Macro Definition Documentation
#define C_ARRAY_ADD | ( | v | ) |
#define FILTER | ( | xm2, | |
xm1, | |||
xp1, | |||
xp2 | |||
) |
Referenced by calc_combed_score().
#define FLAGS AV_OPT_FLAG_VIDEO_PARAM|AV_OPT_FLAG_FILTERING_PARAM |
Definition at line 114 of file vf_fieldmatch.c.
#define HAS_FF_AROUND | ( | p, | |
lz | |||
) |
#define INPUT_CLEANSRC 1 |
Definition at line 43 of file vf_fieldmatch.c.
Referenced by config_output(), fieldmatch_init(), filter_frame(), and request_frame().
#define INPUT_MAIN 0 |
Definition at line 42 of file vf_fieldmatch.c.
Referenced by config_output(), fieldmatch_init(), filter_frame(), and request_frame().
#define LOAD_COMB | ( | mid | ) |
Referenced by checkmm().
#define OFFSET | ( | x | ) | offsetof(FieldMatchContext, x) |
Definition at line 113 of file vf_fieldmatch.c.
#define SLIDING_FRAME_WINDOW | ( | prv, | |
src, | |||
nxt | |||
) |
Referenced by filter_frame().
#define VERTICAL_HALF | ( | y_start, | |
y_end | |||
) |
Referenced by calc_combed_score().
Enumeration Type Documentation
anonymous enum |
Enumerator | |
---|---|
mP | |
mC | |
mN | |
mB | |
mU |
Definition at line 472 of file vf_fieldmatch.c.
enum comb_dbg |
Enumerator | |
---|---|
COMBDBG_NONE | |
COMBDBG_PCN | |
COMBDBG_PCNUB | |
NB_COMBDBG |
Definition at line 68 of file vf_fieldmatch.c.
enum comb_matching_mode |
Enumerator | |
---|---|
COMBMATCH_NONE | |
COMBMATCH_SC | |
COMBMATCH_FULL | |
NB_COMBMATCH |
Definition at line 61 of file vf_fieldmatch.c.
enum fieldmatch_parity |
Enumerator | |
---|---|
FM_PARITY_AUTO | |
FM_PARITY_BOTTOM | |
FM_PARITY_TOP |
Definition at line 45 of file vf_fieldmatch.c.
enum matching_mode |
Enumerator | |
---|---|
MODE_PC | |
MODE_PC_N | |
MODE_PC_U | |
MODE_PC_N_UB | |
MODE_PCN | |
MODE_PCN_UB | |
NB_MODE |
Definition at line 51 of file vf_fieldmatch.c.
Function Documentation
AVFILTER_DEFINE_CLASS | ( | fieldmatch | ) |
|
static |
Definition at line 393 of file vf_fieldmatch.c.
Referenced by build_diff_map().
|
static |
Build a map over which pixels differ a lot/a little.
Definition at line 414 of file vf_fieldmatch.c.
Referenced by compare_fields().
|
static |
Definition at line 195 of file vf_fieldmatch.c.
Referenced by filter_frame().
|
static |
Definition at line 645 of file vf_fieldmatch.c.
Referenced by filter_frame().
|
static |
Definition at line 486 of file vf_fieldmatch.c.
Referenced by filter_frame().
|
static |
Definition at line 864 of file vf_fieldmatch.c.
Referenced by fieldmatch_init().
|
static |
Definition at line 949 of file vf_fieldmatch.c.
|
static |
Definition at line 608 of file vf_fieldmatch.c.
Referenced by create_weave_frame().
|
static |
Definition at line 618 of file vf_fieldmatch.c.
Referenced by filter_frame().
|
static |
Definition at line 895 of file vf_fieldmatch.c.
|
static |
Definition at line 931 of file vf_fieldmatch.c.
Definition at line 185 of file vf_fieldmatch.c.
Referenced by calc_combed_score(), and compare_fields().
|
static |
Definition at line 672 of file vf_fieldmatch.c.
Referenced by fieldmatch_init(), and request_inlink().
|
static |
Definition at line 474 of file vf_fieldmatch.c.
Referenced by compare_fields().
|
static |
Definition at line 160 of file vf_fieldmatch.c.
Referenced by calc_combed_score(), compare_fields(), and copy_fields().
|
static |
Definition at line 155 of file vf_fieldmatch.c.
Referenced by calc_combed_score(), compare_fields(), and copy_fields().
Definition at line 165 of file vf_fieldmatch.c.
Referenced by filter_frame().
|
static |
Definition at line 852 of file vf_fieldmatch.c.
|
static |
Definition at line 836 of file vf_fieldmatch.c.
|
static |
Definition at line 820 of file vf_fieldmatch.c.
Referenced by request_frame().
|
static |
Definition at line 479 of file vf_fieldmatch.c.
Referenced by compare_fields().
Variable Documentation
AVFilter avfilter_vf_fieldmatch |
Definition at line 975 of file vf_fieldmatch.c.
|
static |
Definition at line 116 of file vf_fieldmatch.c.
|
static |
Definition at line 965 of file vf_fieldmatch.c.
Definition at line 669 of file vf_fieldmatch.c.
Referenced by filter_frame().
Definition at line 670 of file vf_fieldmatch.c.
Referenced by filter_frame().
Generated on Fri Mar 28 2025 06:53:15 for FFmpeg by
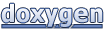