svcore
1.9
|
ModelDataTableModel.cpp
Go to the documentation of this file.
213 // cerr << "Current row changed from " << prevCurrent << " to " << current << " for underlying row " << m_currentRow << endl;
417 // cerr << "Current row changed from " << prevCurrent << " to " << current << " for underlying row " << m_currentRow << endl;
void modelChangedWithin(ModelId, sv_frame_t, sv_frame_t)
Definition: ModelDataTableModel.cpp:238
ModelDataTableModel(ModelId modelId)
Definition: ModelDataTableModel.cpp:25
int getUnsorted(int row) const
Definition: ModelDataTableModel.cpp:286
bool removeRow(int row, const QModelIndex &parent=QModelIndex())
Definition: ModelDataTableModel.cpp:89
Definition: TabularModel.h:57
QModelIndex parent(const QModelIndex &index) const override
Definition: ModelDataTableModel.cpp:134
QVariant headerData(int section, Qt::Orientation orientation, int role=Qt::DisplayRole) const override
Definition: ModelDataTableModel.cpp:113
Definition: TabularModel.h:58
QModelIndex getModelIndexForFrame(sv_frame_t frame) const
Definition: ModelDataTableModel.cpp:159
int rowCount(const QModelIndex &parent=QModelIndex()) const override
Definition: ModelDataTableModel.cpp:140
Definition: TabularModel.h:58
int columnCount(const QModelIndex &parent=QModelIndex()) const override
Definition: ModelDataTableModel.cpp:150
void resortAlphabetical() const
Definition: ModelDataTableModel.cpp:374
bool insertRow(int row, const QModelIndex &parent=QModelIndex())
Definition: ModelDataTableModel.cpp:73
QModelIndex index(int row, int column, const QModelIndex &parent=QModelIndex()) const override
Definition: ModelDataTableModel.cpp:128
QVariant data(const QModelIndex &index, int role) const override
Definition: ModelDataTableModel.cpp:45
bool setData(const QModelIndex &index, const QVariant &value, int role) override
Definition: ModelDataTableModel.cpp:56
std::shared_ptr< TabularModel > getTabularModel() const
Definition: ModelDataTableModel.h:80
void setCurrentRow(int row)
Definition: ModelDataTableModel.cpp:405
Qt::ItemFlags flags(const QModelIndex &index) const override
Definition: ModelDataTableModel.cpp:105
void resortNumeric() const
Definition: ModelDataTableModel.cpp:350
Definition: Command.h:26
sv_frame_t getFrameForModelIndex(const QModelIndex &) const
Definition: ModelDataTableModel.cpp:168
void addCommand(Command *)
QModelIndex findText(QString text) const
Definition: ModelDataTableModel.cpp:176
void sort(int column, Qt::SortOrder order=Qt::AscendingOrder) override
Definition: ModelDataTableModel.cpp:201
virtual ~ModelDataTableModel()
Definition: ModelDataTableModel.cpp:40
Definition: ById.h:115
void currentChanged(const QModelIndex &)
Generated by
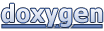