svcore
1.9
|
#include <ModelDataTableModel.h>
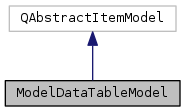
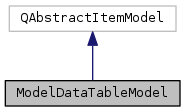
Signals | |
void | frameSelected (int) |
void | addCommand (Command *) |
void | currentChanged (const QModelIndex &) |
void | modelRemoved () |
Public Member Functions | |
ModelDataTableModel (ModelId modelId) | |
virtual | ~ModelDataTableModel () |
QVariant | data (const QModelIndex &index, int role) const override |
bool | setData (const QModelIndex &index, const QVariant &value, int role) override |
bool | insertRow (int row, const QModelIndex &parent=QModelIndex()) |
bool | removeRow (int row, const QModelIndex &parent=QModelIndex()) |
Qt::ItemFlags | flags (const QModelIndex &index) const override |
QVariant | headerData (int section, Qt::Orientation orientation, int role=Qt::DisplayRole) const override |
QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const override |
QModelIndex | parent (const QModelIndex &index) const override |
int | rowCount (const QModelIndex &parent=QModelIndex()) const override |
int | columnCount (const QModelIndex &parent=QModelIndex()) const override |
QModelIndex | getModelIndexForFrame (sv_frame_t frame) const |
sv_frame_t | getFrameForModelIndex (const QModelIndex &) const |
void | sort (int column, Qt::SortOrder order=Qt::AscendingOrder) override |
QModelIndex | findText (QString text) const |
void | setCurrentRow (int row) |
int | getCurrentRow () const |
Protected Types | |
typedef std::vector< int > | RowList |
Protected Slots | |
void | modelChanged (ModelId) |
void | modelChangedWithin (ModelId, sv_frame_t, sv_frame_t) |
Protected Member Functions | |
std::shared_ptr< TabularModel > | getTabularModel () const |
int | getSorted (int row) const |
int | getUnsorted (int row) const |
void | resort () const |
void | resortNumeric () const |
void | resortAlphabetical () const |
void | clearSort () |
Protected Attributes | |
ModelId | m_model |
int | m_sortColumn |
Qt::SortOrder | m_sortOrdering |
int | m_currentRow |
RowList | m_sort |
RowList | m_rsort |
Detailed Description
Definition at line 31 of file ModelDataTableModel.h.
Member Typedef Documentation
|
protected |
Definition at line 88 of file ModelDataTableModel.h.
Constructor & Destructor Documentation
ModelDataTableModel::ModelDataTableModel | ( | ModelId | modelId | ) |
Definition at line 25 of file ModelDataTableModel.cpp.
References TypedById< Item, Id >::get(), modelChanged(), and modelChangedWithin().
|
virtual |
Definition at line 40 of file ModelDataTableModel.cpp.
Member Function Documentation
|
override |
Definition at line 45 of file ModelDataTableModel.cpp.
References getTabularModel(), and getUnsorted().
|
override |
Definition at line 56 of file ModelDataTableModel.cpp.
References addCommand(), getTabularModel(), and getUnsorted().
bool ModelDataTableModel::insertRow | ( | int | row, |
const QModelIndex & | parent = QModelIndex() |
||
) |
Definition at line 73 of file ModelDataTableModel.cpp.
References addCommand(), getTabularModel(), and getUnsorted().
bool ModelDataTableModel::removeRow | ( | int | row, |
const QModelIndex & | parent = QModelIndex() |
||
) |
Definition at line 89 of file ModelDataTableModel.cpp.
References addCommand(), getTabularModel(), and getUnsorted().
|
override |
Definition at line 105 of file ModelDataTableModel.cpp.
|
override |
Definition at line 113 of file ModelDataTableModel.cpp.
References getTabularModel().
|
override |
Definition at line 128 of file ModelDataTableModel.cpp.
|
override |
Definition at line 134 of file ModelDataTableModel.cpp.
|
override |
Definition at line 140 of file ModelDataTableModel.cpp.
References getTabularModel().
Referenced by findText(), getSorted(), getUnsorted(), modelChanged(), and modelChangedWithin().
|
override |
Definition at line 150 of file ModelDataTableModel.cpp.
References getTabularModel().
Referenced by findText(), and modelChanged().
QModelIndex ModelDataTableModel::getModelIndexForFrame | ( | sv_frame_t | frame | ) | const |
Definition at line 159 of file ModelDataTableModel.cpp.
References getSorted(), and getTabularModel().
Referenced by modelChangedWithin().
sv_frame_t ModelDataTableModel::getFrameForModelIndex | ( | const QModelIndex & | index | ) | const |
Definition at line 168 of file ModelDataTableModel.cpp.
References getTabularModel(), and getUnsorted().
|
override |
Definition at line 201 of file ModelDataTableModel.cpp.
References clearSort(), currentChanged(), getCurrentRow(), m_sortColumn, and m_sortOrdering.
QModelIndex ModelDataTableModel::findText | ( | QString | text | ) | const |
Definition at line 176 of file ModelDataTableModel.cpp.
References columnCount(), getCurrentRow(), getTabularModel(), getUnsorted(), rowCount(), and TabularModel::SortAlphabetical.
void ModelDataTableModel::setCurrentRow | ( | int | row | ) |
Definition at line 405 of file ModelDataTableModel.cpp.
References getUnsorted(), and m_currentRow.
int ModelDataTableModel::getCurrentRow | ( | ) | const |
Definition at line 399 of file ModelDataTableModel.cpp.
References getSorted(), and m_currentRow.
Referenced by findText(), and sort().
|
signal |
|
signal |
Referenced by insertRow(), removeRow(), and setData().
|
signal |
Referenced by sort().
|
signal |
|
protectedslot |
Definition at line 220 of file ModelDataTableModel.cpp.
References clearSort(), columnCount(), rowCount(), and SVDEBUG.
Referenced by ModelDataTableModel().
|
protectedslot |
Definition at line 238 of file ModelDataTableModel.cpp.
References clearSort(), getModelIndexForFrame(), rowCount(), and SVDEBUG.
Referenced by ModelDataTableModel().
|
inlineprotected |
Definition at line 80 of file ModelDataTableModel.h.
References m_model.
Referenced by columnCount(), data(), findText(), getFrameForModelIndex(), getModelIndexForFrame(), getSorted(), getUnsorted(), headerData(), insertRow(), removeRow(), resort(), resortAlphabetical(), resortNumeric(), rowCount(), and setData().
|
protected |
Definition at line 258 of file ModelDataTableModel.cpp.
References getTabularModel(), m_sort, m_sortColumn, m_sortOrdering, resort(), and rowCount().
Referenced by getCurrentRow(), and getModelIndexForFrame().
|
protected |
Definition at line 286 of file ModelDataTableModel.cpp.
References getTabularModel(), m_rsort, m_sort, m_sortColumn, m_sortOrdering, resort(), and rowCount().
Referenced by data(), findText(), getFrameForModelIndex(), insertRow(), removeRow(), setCurrentRow(), and setData().
|
protected |
Definition at line 316 of file ModelDataTableModel.cpp.
References getTabularModel(), m_rsort, m_sort, m_sortColumn, resortAlphabetical(), resortNumeric(), and TabularModel::SortNumeric.
Referenced by getSorted(), and getUnsorted().
|
protected |
Definition at line 350 of file ModelDataTableModel.cpp.
References getTabularModel(), m_rsort, m_sortColumn, and TabularModel::SortRole.
Referenced by resort().
|
protected |
Definition at line 374 of file ModelDataTableModel.cpp.
References getTabularModel(), m_rsort, m_sortColumn, and TabularModel::SortRole.
Referenced by resort().
|
protected |
Definition at line 411 of file ModelDataTableModel.cpp.
References m_sort.
Referenced by modelChanged(), modelChangedWithin(), and sort().
Member Data Documentation
|
protected |
Definition at line 84 of file ModelDataTableModel.h.
Referenced by getTabularModel().
|
protected |
Definition at line 85 of file ModelDataTableModel.h.
Referenced by getSorted(), getUnsorted(), resort(), resortAlphabetical(), resortNumeric(), and sort().
|
protected |
Definition at line 86 of file ModelDataTableModel.h.
Referenced by getSorted(), getUnsorted(), and sort().
|
protected |
Definition at line 87 of file ModelDataTableModel.h.
Referenced by getCurrentRow(), and setCurrentRow().
|
mutableprotected |
Definition at line 89 of file ModelDataTableModel.h.
Referenced by clearSort(), getSorted(), getUnsorted(), and resort().
|
mutableprotected |
Definition at line 90 of file ModelDataTableModel.h.
Referenced by getUnsorted(), resort(), resortAlphabetical(), and resortNumeric().
The documentation for this class was generated from the following files:
Generated by
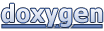