Bela
|
Classes | |
struct | BelaContext |
Structure holding current audio and sensor settings and pointers to data buffers. More... | |
Functions | |
bool | setup (BelaContext *context, void *userData) |
User-defined initialisation function which runs before audio rendering begins. More... | |
void | render (BelaContext *context, void *userData) |
User-defined callback function to process audio and sensor data. More... | |
void | cleanup (BelaContext *context, void *userData) |
User-defined cleanup function which runs when the program finishes. More... | |
Variables | |
int | gShouldStop |
Detailed Description
These three functions must be implemented by the developer in every Bela program. Typically they appear in their own .cpp source file.
Function Documentation
bool setup | ( | BelaContext * | context, |
void * | userData | ||
) |
User-defined initialisation function which runs before audio rendering begins.
This function runs once at the beginning of the program, after most of the system initialisation has begun but before audio rendering starts. Use it to prepare any memory or resources that will be needed in render().
- Parameters
-
context Data structure holding information on sample rates, numbers of channels, frame sizes and other state. Note: the buffers for audio, analog and digital data will not yet be available to use. Do not attempt to read or write audio or sensor data in setup(). userData An opaque pointer to an optional user-defined data structure. Whatever is passed as the second argument to Bela_initAudio() will appear here.
- Returns
- true on success, or false if an error occurred. If no initialisation is required, setup() should return true.
- Examples:
- analog-input/render.cpp, analog-output/render.cpp, capacitive-touch/render.cpp, digital-input/render.cpp, digital-output/render.cpp, FFT-audio-in/render.cpp, FFT-phase-vocoder/render.cpp, filter-FIR/render.cpp, filter-IIR/render.cpp, logging-sensors/render.cpp, minimal/render.cpp, OSC/render.cpp, oscillator-bank/render.cpp, passthrough/render.cpp, samples/render.cpp, scope-analog/render.cpp, scope/render.cpp, sinetone/render.cpp, tremolo/render.cpp, userdata/render.cpp, and write-file/render.cpp.
void render | ( | BelaContext * | context, |
void * | userData | ||
) |
User-defined callback function to process audio and sensor data.
This function is called regularly by the system every time there is a new block of audio and/or sensor data to process. Your code should process the requested samples of data, store the results within context
, and return.
- Parameters
-
context Data structure holding buffers for audio, analog and digital data. The structure also holds information on numbers of channels, frame sizes and sample rates, which are guaranteed to remain the same throughout the program and to match what was passed to setup(). userData An opaque pointer to an optional user-defined data structure. Will be the same as the userData
parameter passed to setup().
Checking offset between analog and digital how it should be : The PRU loop does the following (the loop runs at 88.2kHz):
- Read/write audio sample (once for the left channel, once for the right channel)
- Write DAC 0 or 0/2 or 0/2/4/6
- Read ADC 0 or 0/2 or 0/2/4/6, 2 samples (@176.4) older than NOW
- /During/ the line above, every two loops we also Read/Write GPIO, therefore reading on ADC 0/2/4/6 a value that is being output from GPIO will lead to undefined results
- Write DAC 1 or 1/3 or 1/3/5/7
- Read ADC 1 or 1/3 or 1/3/5/7, 2 samples (@176.4) older than NOW
- Examples:
- analog-input/render.cpp, analog-output/render.cpp, capacitive-touch/render.cpp, digital-input/render.cpp, digital-output/render.cpp, FFT-audio-in/render.cpp, FFT-phase-vocoder/render.cpp, filter-FIR/render.cpp, filter-IIR/render.cpp, logging-sensors/render.cpp, minimal/render.cpp, OSC/render.cpp, oscillator-bank/render.cpp, passthrough/render.cpp, samples/render.cpp, scope-analog/render.cpp, scope/render.cpp, sinetone/render.cpp, tremolo/render.cpp, userdata/render.cpp, and write-file/render.cpp.
void cleanup | ( | BelaContext * | context, |
void * | userData | ||
) |
User-defined cleanup function which runs when the program finishes.
This function is called by the system once after audio rendering has finished, before the program quits. Use it to release any memory allocated in setup() and to perform any other required cleanup. If no initialisation is performed in setup(), then this function will usually be empty.
- Parameters
-
context Data structure holding information on sample rates, numbers of channels, frame sizes and other state. Note: the buffers for audio, analog and digital data will no longer be available to use. Do not attempt to read or write audio or sensor data in cleanup(). userData An opaque pointer to an optional user-defined data structure. Will be the same as the userData
parameter passed to setup() and render().
- Examples:
- analog-input/render.cpp, analog-output/render.cpp, capacitive-touch/render.cpp, digital-input/render.cpp, digital-output/render.cpp, FFT-audio-in/render.cpp, FFT-phase-vocoder/render.cpp, filter-FIR/render.cpp, filter-IIR/render.cpp, logging-sensors/render.cpp, minimal/render.cpp, OSC/render.cpp, oscillator-bank/render.cpp, passthrough/render.cpp, samples/render.cpp, scope-analog/render.cpp, scope/render.cpp, sinetone/render.cpp, tremolo/render.cpp, userdata/render.cpp, and write-file/render.cpp.
Variable Documentation
int gShouldStop |
Flag that indicates when the audio will stop. Threads can poll this variable to indicate when they should stop. Additionally, a program can set this to true
to indicate that audio processing should terminate. Calling Bela_stopAudio() has the effect of setting this to true
.
- Examples:
- filter-FIR/render.cpp, filter-IIR/render.cpp, and samples/render.cpp.
Generated on Wed May 28 2025 06:27:57 for Bela by
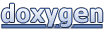