Bela
|
Switching an LED on and off
This example brings together digital input and digital output. The program will read a button and turn the LED on and off according to the state of the button.
- connect an LED in series with a 470ohm resistor between P8_07 and ground.
- connect a 1k resistor to P9_03 (+3.3V),
- connect the other end of the resistor to both a button and P8_08
- connect the other end of the button to ground.
You will notice that the LED will normally stay on and will turn off as long as the button is pressed. This is due to the fact that the LED is set to the same value read at input P8_08. When the button is not pressed, P8_08 is HIGH
and so P8_07 is set to HIGH
as well, so that the LED conducts and emits light. When the button is pressed, P8_08 goes LOW
and P8_07 is set to LOW
, turning off the LED.
Note that there are two ways of specifying the digital pin: using the GPIO label (e.g. P8_07
), or using the digital IO index (e.g. 0)
As an exercise try and change the code so that the LED only turns on when the button is pressed.
Generated on Thu Jun 19 2025 06:28:07 for Bela by
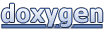