Bela
|
Main Bela public API. More...
#include <stdint.h>
#include <unistd.h>
#include <rtdk.h>
#include "digital_gpio_mapping.h"
#include <GPIOcontrol.h>
#include <Utilities.h>
Include dependency graph for Bela.h:

This graph shows which files directly or indirectly include this file:

Go to the source code of this file.
Classes | |
struct | BelaInitSettings |
Structure containing initialisation parameters for the real-time audio control system. More... | |
struct | BelaContext |
Structure holding current audio and sensor settings and pointers to data buffers. More... | |
Macros | |
#define | BELA_AUDIO_PRIORITY 95 |
#define | DEFAULT_DAC_LEVEL 0.0 |
#define | DEFAULT_ADC_LEVEL -6.0 |
#define | DEFAULT_PGA_GAIN 16 |
#define | DEFAULT_HP_LEVEL -6.0 |
#define | BELA_FLAG_INTERLEAVED (1 << 0) |
#define | BELA_FLAG_ANALOG_OUTPUTS_PERSIST (1 << 1) |
Typedefs | |
typedef void * | AuxiliaryTask |
Functions | |
bool | setup (BelaContext *context, void *userData) |
User-defined initialisation function which runs before audio rendering begins. More... | |
void | render (BelaContext *context, void *userData) |
User-defined callback function to process audio and sensor data. More... | |
void | cleanup (BelaContext *context, void *userData) |
User-defined cleanup function which runs when the program finishes. More... | |
void | Bela_defaultSettings (BelaInitSettings *settings) |
Initialise the data structure containing settings for Bela. More... | |
int | Bela_getopt_long (int argc, char *argv[], const char *customShortOptions, const struct option *customLongOptions, BelaInitSettings *settings) |
Get long options from command line argument list, including Bela standard options. More... | |
void | Bela_usage () |
Print usage information for Bela standard options. More... | |
void | Bela_setVerboseLevel (int level) |
Set level of verbose (debugging) printing. More... | |
int | Bela_initAudio (BelaInitSettings *settings, void *userData) |
Initialise audio and sensor rendering environment. More... | |
int | Bela_startAudio () |
Begin processing audio and sensor data. More... | |
int | Bela_startAuxiliaryTask (AuxiliaryTask it) |
Stop processing audio and sensor data. More... | |
void | Bela_stopAudio () |
void | Bela_cleanupAudio () |
Clean up resources from audio and sensor processing. More... | |
int | Bela_setDACLevel (float decibels) |
Set the level of the audio DAC. More... | |
int | Bela_setADCLevel (float decibels) |
Set the level of the audio ADC. More... | |
int | Bela_setPgaGain (float decibels, int channel) |
Set the gain of the audio preamplifier. More... | |
int | Bela_setHeadphoneLevel (float decibels) |
Set the level of the onboard headphone amplifier. More... | |
int | Bela_muteSpeakers (int mute) |
Mute or unmute the onboard speaker amplifiers. More... | |
AuxiliaryTask | Bela_createAuxiliaryTask (void(*functionToCall)(void *), int priority, const char *name, void *args, bool autoSchedule=false) |
Create a new auxiliary task. More... | |
AuxiliaryTask | Bela_createAuxiliaryTask (void(*functionToCall)(void), int priority, const char *name, bool autoSchedule=false) |
void | Bela_scheduleAuxiliaryTask (AuxiliaryTask task) |
Run an auxiliary task which has previously been created. More... | |
void | Bela_autoScheduleAuxiliaryTasks () |
Variables | |
int | gShouldStop |
Detailed Description
Main Bela public API.
Central control code for hard real-time audio on BeagleBone Black using PRU and Xenomai Linux extensions. This code began as part of the Hackable Instruments project (EPSRC) at Queen Mary University of London, 2013-14.
(c) 2014-15 Andrew McPherson, Victor Zappi and Giulio Moro, Queen Mary University of London
Macro Definition Documentation
#define BELA_FLAG_INTERLEAVED (1 << 0) |
Flag for BelaContext. If set, indicates the audio and analog buffers are interleaved.
#define BELA_FLAG_ANALOG_OUTPUTS_PERSIST (1 << 1) |
Flag for BelaContext. If set, indicates analog outputs persist for future frames.
Generated on Fri Aug 1 2025 06:28:13 for Bela by
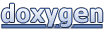